Python Exercises: Two strings contain three letters at the same index
Python String: Exercise-107 with Solution
Write a Python program that takes two strings. Count the number of times each string contains the same three letters at the same index.
Sample Data:
(“Red RedGreen”) -> 1
(“Red White Red White) -> 7
(“Red White White Red”) -> 0
Sample Solution-1:
Python Code:
# Define a function to check if two strings contain the same three letters at the same index
def test(text1, text2):
# Initialize a counter variable to count the occurrences
ctr = 0
# Iterate through the range of indices up to the third-to-last index
for i in range(len(text1) - 2):
# Check if the substring of length 3 from both strings at the current index is the same
if text1[i:i+3] == text2[i:i+3]:
# If true, increment the counter
ctr += 1
# Return the count of occurrences
return ctr
# Initialize two strings
text1 = "Red"
text2 = "RedGreen"
# Print the original strings
print("Original strings:", text1, text2)
# Print a message indicating the check for the same three letters at the same index
print("Check said two strings contain three letters at the same index:")
# Call the function to check and print the result
print(test(text1, text2))
# Repeat the process with different strings
text1 = "Red White"
text2 = "Red White"
# Print the original strings
print("\nOriginal strings:", text1, text2)
# Print a message indicating the check for the same three letters at the same index
print("Check said two strings contain three letters at the same index:")
# Call the function to check and print the result
print(test(text1, text2))
# Repeat the process with another set of different strings
text1 = "Red White"
text2 = "White Red"
# Print the original strings
print("\nOriginal strings:", text1, text2)
# Print a message indicating the check for the same three letters at the same index
print("Check said two strings contain three letters at the same index:")
# Call the function to check and print the result
print(test(text1, text2))
Sample Output:
Original strings: Red RedGreen Check said two strings contain three letters at the same index: 1 Original strings: Red White Red White Check said two strings contain three letters at the same index: 7 Original strings: Red White White Red Check said two strings contain three letters at the same index: 0
Flowchart:
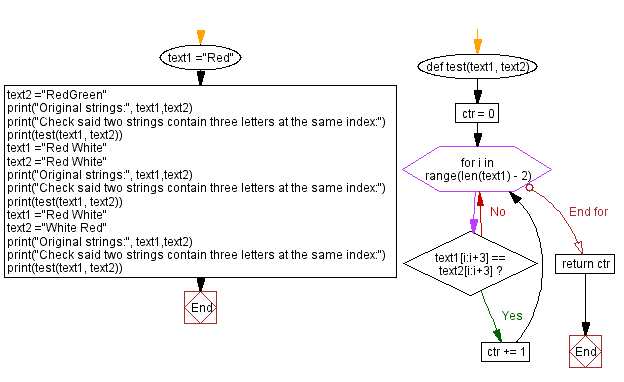
Sample Solution-2:
Python Code:
# Define a function to count the occurrences of three consecutive letters at the same index in two strings
def test(text1, text2):
# Use a list comprehension to create a list of 1s for each match, then sum the list to get the count
return sum([1 for i in range(len(text1)-2) if text1[i:i+3] == text2[i:i+3]])
# Initialize two strings
text1 = "Red"
text2 = "RedGreen"
# Print the original strings
print("Original strings:", text1, text2)
# Print a message indicating the check for the same three letters at the same index
print("Check said two strings contain three letters at the same index:")
# Call the function to check and print the result
print(test(text1, text2))
# Repeat the process with different strings
text1 = "Red White"
text2 = "Red White"
# Print the original strings
print("\nOriginal strings:", text1, text2)
# Print a message indicating the check for the same three letters at the same index
print("Check said two strings contain three letters at the same index:")
# Call the function to check and print the result
print(test(text1, text2))
# Repeat the process with another set of different strings
text1 = "Red White"
text2 = "White Red"
# Print the original strings
print("\nOriginal strings:", text1, text2)
# Print a message indicating the check for the same three letters at the same index
print("Check said two strings contain three letters at the same index:")
# Call the function to check and print the result
print(test(text1, text2))
Sample Output:
Original strings: Red RedGreen Check said two strings contain three letters at the same index: 1 Original strings: Red White Red White Check said two strings contain three letters at the same index: 7 Original strings: Red White White Red Check said two strings contain three letters at the same index: 0
Flowchart:
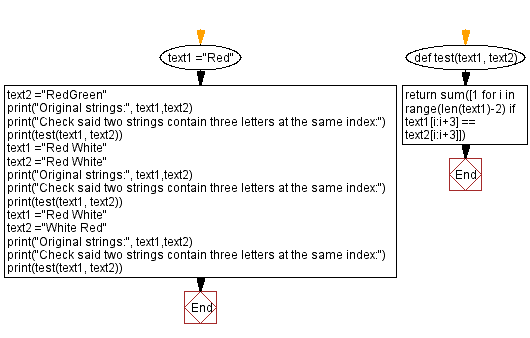
Python Code Editor:
Previous Python Exercise: Replace repeated characters with single letters.
Next Python Exercise: Hash elements.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-107.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics