Python: Remove the characters which have odd index values of a given string
Python String: Exercise-11 with Solution
Write a Python program to remove characters that have odd index values in a given string.
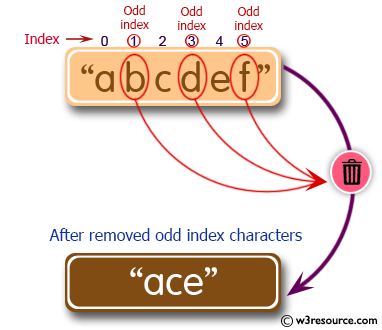
Sample Solution:
Python Code:
# Define a function named odd_values_string that takes one argument, 'str'.
def odd_values_string(str):
# Initialize an empty string 'result' to store characters with odd indices.
result = ""
# Iterate through the indices (i) of the characters in the input string 'str'.
for i in range(len(str)):
# Check if the index (i) is even (i.e., has a remainder of 0 when divided by 2).
if i % 2 == 0:
# If the index is even, append the character at that index to the 'result' string.
result = result + str[i]
# Return the 'result' string containing characters with odd indices.
return result
# Call the odd_values_string function with different input strings and print the results.
print(odd_values_string('abcdef')) # Output: 'ace'
print(odd_values_string('python')) # Output: 'pto'
Sample Output:
ace pto
Flowchart:
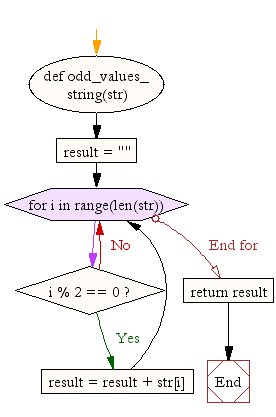
Python Code Editor:
Previous: Write a Python program to change a given string to a new string where the first and last chars have been exchanged.
Next: Write a Python program to count the occurrences of each word in a given sentence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-11.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics