Python Exercises: Insert space before capital letters in word
Python String: Exercise-110 with Solution
Write a Python program to insert space before every capital letter appears in a given word.
Sample Data:
("PythonExercises") -> "Python Exercises"
("Python") -> "Python"
(“PythonExercisesPracticeSolution”) -> “Python Exercises Practice Solution”
Sample Solution:
Python Code:
# Define a function to insert a space before capital letters in a given word
def test(word):
result = ""
# Iterate through each character in the word
for i in word:
# Check if the character is uppercase
if i.isupper():
# Concatenate a space and the uppercase version of the character to the result
result = result + " " + i.upper()
else:
# Concatenate the character to the result
result = result + i
# Remove the leading space from the result and return
return result[1:]
# Initialize a string representing a word
word = "PythonExercises"
# Print the original word
print("Original Word:", word)
# Print a message indicating the insertion of space before capital letters
print("Insert space before capital letters in the said word:")
# Call the function to modify the word and print the result
print(test(word))
# Repeat the process with a different word
word = "Python"
# Print the original word
print("\nOriginal Word:", word)
# Print a message indicating the insertion of space before capital letters
print("Insert space before capital letters in the said word:")
# Call the function to modify the word and print the result
print(test(word))
# Repeat the process with a different word
word = "PythonExercisesPracticeSolution"
# Print the original word
print("\nOriginal Word:", word)
# Print a message indicating the insertion of space before capital letters
print("Insert space before capital letters in the said word:")
# Call the function to modify the word and print the result
print(test(word))
Sample Output:
Original Word: PythonExercises Insert space before capital letters in the said word: Python Exercises Original Word: Python Insert space before capital letters in the said word: Python Original Word: PythonExercisesPracticeSolution Insert space before capital letters in the said word: Python Exercises Practice Solution
Flowchart:
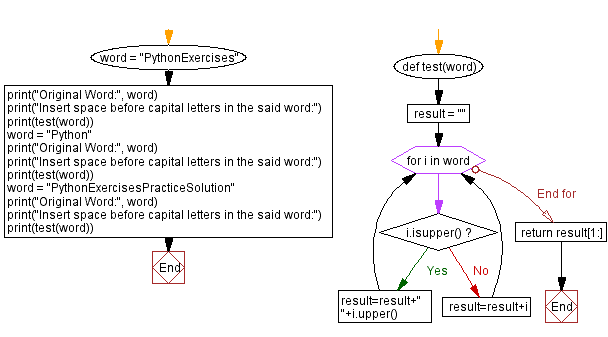
Python Code Editor:
Previous Python Exercise: Count the number of leap years within the range.
Next Python Exercise: Alphabet position in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-110.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics