Python Exercises: Alphabet position in a string
Replace chars with position numbers.
Write a Python program that takes a string and replaces all the characters with their respective numbers.
Sample Data:
("Python") -> "16 25 20 8 15 14"
("Java") -> "10 1 22 1"
(“Python Tutorial”) -> "16 25 20 8 15 14 20 21 20 15 18 9 1 12"
Sample Solution-1:
Python Code:
# Define a function to return the alphabet position of each letter in a given string
def test(text):
# Use a list comprehension to generate a list of alphabet positions for each alphabetic character
# Convert each character to lowercase and use the ord function to get its ASCII value, then subtract 96
return ' '.join(str(ord(i)-96) for i in text.lower() if i.isalpha())
# Initialize a string representing a word
word = "Python"
# Print the original word
print("Original Word:", word)
# Print a message indicating the alphabet position in the said string
print("Alphabet position in the said string:")
# Call the function to get the alphabet positions and print the result
print(test(word))
# Repeat the process with a different word
word = "Java"
# Print the original word
print("\nOriginal Word:", word)
# Print a message indicating the alphabet position in the said string
print("Alphabet position in the said string:")
# Call the function to get the alphabet positions and print the result
print(test(word))
# Repeat the process with a different word
word = "Python Tutorial"
# Print the original word
print("\nOriginal Word:", word)
# Print a message indicating the alphabet position in the said string
print("Alphabet position in the said string:")
# Call the function to get the alphabet positions and print the result
print(test(word))
Sample Output:
Original Word: Python Alphabet position in the said string: 16 25 20 8 15 14 Original Word: Java Alphabet position in the said string: 10 1 22 1 Original Word: Python Tutorial Alphabet position in the said string: 16 25 20 8 15 14 20 21 20 15 18 9 1 12
Flowchart:
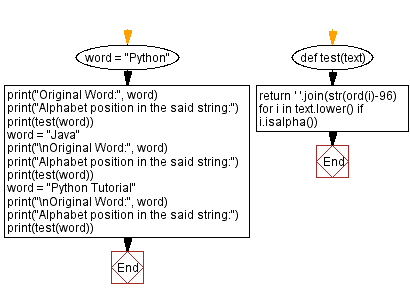
Sample Solution-2:
Python Code:
# Define a function to return the alphabet position of each letter in a given string
def test(text):
# Use a list comprehension to generate a list of alphabet positions for each alphabetic character
# Convert each character to lowercase and use the ord function to get its ASCII value,
# then subtract the ASCII value of 'a' and add 1
return ' '.join(str(ord(el) - ord('a') + 1) for el in text.lower() if el.isalpha())
# Initialize a string representing a word
word = "Python"
# Print the original word
print("Original Word:", word)
# Print a message indicating the alphabet position in the said string
print("Alphabet position in the said string:")
# Call the function to get the alphabet positions and print the result
print(test(word))
# Repeat the process with a different word
word = "Java"
# Print the original word
print("\nOriginal Word:", word)
# Print a message indicating the alphabet position in the said string
print("Alphabet position in the said string:")
# Call the function to get the alphabet positions and print the result
print(test(word))
# Repeat the process with a different word
word = "Python Tutorial"
# Print the original word
print("\nOriginal Word:", word)
# Print a message indicating the alphabet position in the said string
print("Alphabet position in the said string:")
# Call the function to get the alphabet positions and print the result
print(test(word))
Sample Output:
Original Word: Python Alphabet position in the said string: 16 25 20 8 15 14 Original Word: Java Alphabet position in the said string: 10 1 22 1 Original Word: Python Tutorial Alphabet position in the said string: 16 25 20 8 15 14 20 21 20 15 18 9 1 12
Flowchart:
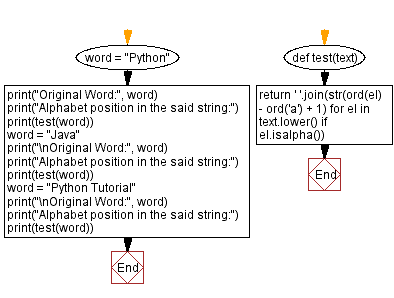
For more Practice: Solve these Related Problems:
- Write a Python program to convert each character in a string to its alphabetical position number and join them with spaces.
- Write a Python program to iterate over a string and replace each letter with its corresponding position in the alphabet using ord().
- Write a Python program to create a function that maps each character of a string to a number, preserving non-alphabetic characters as is.
- Write a Python program to use list comprehension to generate a string of numbers corresponding to each character’s position in the alphabet.
Go to:
Previous Python Exercise: Insert space before capital letters in word.
Next Python Exercise: Calculate the sum of two numbers given as strings.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.