Python Exercises: Calculate the sum of two numbers given as strings
Python String: Exercise-112 with Solution
Write a Python program to calculate the sum of two numbers given as strings. Return the result in the same string representation.
Sample Data:
( “234242342341”, “2432342342”) -> “236674684683”
( “”, “2432342342”) -> False
( “1000”, “10”) -> “1010”
Sample Solution-1:
Python Code:
# Define a function to add two numbers represented as strings
def test(x, y):
# Check if either x or y is an empty string, return False if true
if (x == "" or y == ""):
return False
# Determine the maximum length between x and y
m = max(len(x), len(y))
c = 0 # Initialize carry to 0
result = '' # Initialize the result string
# Iterate through the digits of x and y from right to left
for x, y in zip(x.rjust(m, '0')[::-1], y.rjust(m, '0')[::-1]):
# Add the digits and the carry
s = int(x) + int(y) + c
# Update the carry for the next iteration
c = 1 if s > 9 else 0
# Append the last digit of the sum to the result
result += str(s)[-1]
# Add the final carry to the result if it is 1
result = result + str(c) if c == 1 else result
# Reverse the result and return
return result[::-1]
# Initialize two string numbers
n1 ="234242342341"
n2 ="2432342342"
# Print the original string numbers
print("Original string numbers:", n1, n2)
# Print a message indicating the addition of the two numbers
print("Check if said two strings represent numbers and add them:")
# Call the function to add the numbers and print the result
print(test(n1, n2))
# Repeat the process with different sets of string numbers
n1 =""
n2 ="2432342342"
print("\nOriginal string numbers:", n1, n2)
print("Check if said two strings represent numbers and add them:")
print(test(n1, n2))
n1 ="1000"
n2 ="0"
print("\nOriginal string numbers:", n1, n2)
print("Check if said two strings represent numbers and add them:")
print(test(n1, n2))
n1 ="1000"
n2 ="10"
print("\nOriginal string numbers:", n1, n2)
print("Check if said two strings represent numbers and add them:")
print(test(n1, n2))
Sample Output:
Original string numbers: 234242342341 2432342342 Check said two strings contain three letters at the same index: 236674684683 Original string numbers: 2432342342 Check said two strings contain three letters at the same index: False Original string numbers: 1000 0 Check said two strings contain three letters at the same index: 1000 Original string numbers: 1000 10 Check said two strings contain three letters at the same index: 1010
Flowchart:
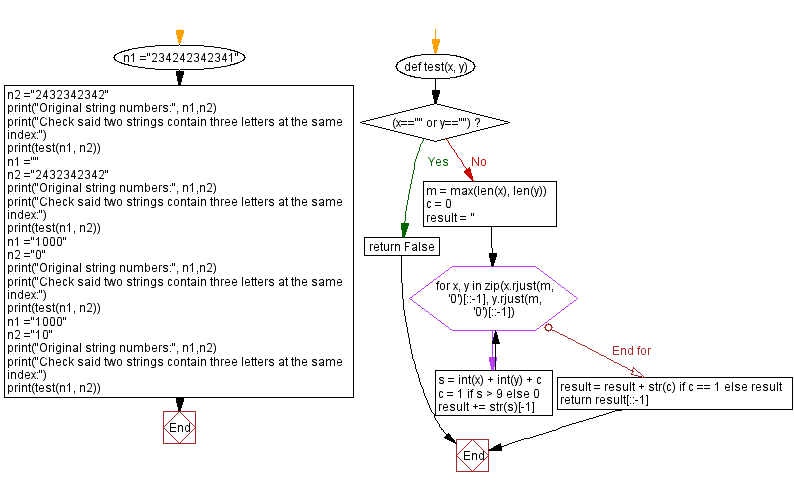
Sample Solution-2:
Python Code:
# Define a function to add two numbers represented as strings
def test(x, y):
# Check if either x or y is an empty string, return False if true
if (x == "" or y == ""):
return False
# Convert the string representations of numbers to integers, add them, and convert back to string
return str(int(x) + int(y))
# Initialize two string numbers
n1 ="234242342341"
n2 ="2432342342"
# Print the original string numbers
print("Original string numbers:", n1, n2)
# Print a message indicating the addition of the two numbers
print("Calculate the sum of two said numbers given as strings:")
# Call the function to add the numbers and print the result
print(test(n1, n2))
# Repeat the process with different sets of string numbers
n1 =""
n2 ="2432342342"
print("\nOriginal string numbers:", n1, n2)
print("Calculate the sum of two said numbers given as strings:")
print(test(n1, n2))
n1 ="1000"
n2 ="0"
print("\nOriginal string numbers:", n1, n2)
print("Calculate the sum of two said numbers given as strings:")
print(test(n1, n2))
n1 ="1000"
n2 ="10"
print("\nOriginal string numbers:", n1, n2)
print("Calculate the sum of two said numbers given as strings:")
print(test(n1, n2))
Sample Output:
Original string numbers: 234242342341 2432342342 Calculate the sum of two said numbers given as strings: 236674684683 Original string numbers: 2432342342 Calculate the sum of two said numbers given as strings: False Original string numbers: 1000 0 Calculate the sum of two said numbers given as strings: 1000 Original string numbers: 1000 10 Calculate the sum of two said numbers given as strings: 1010
Flowchart:
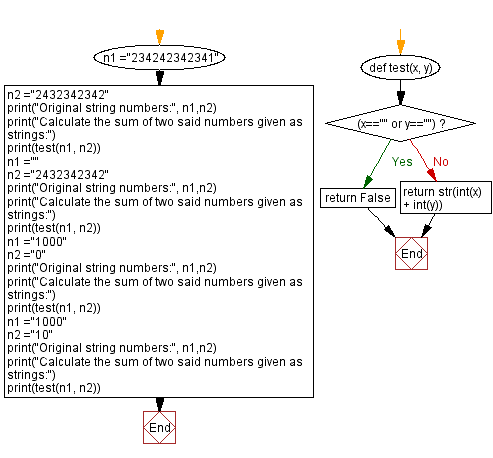
Python Code Editor:
Previous Python Exercise: Alphabet position in a string.
Next Python Exercise: Sort a string based on its first character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-112.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics