Python Exercises: Sort a string based on its first character
Python String: Exercise-113 with Solution
Write a Python program that returns a string sorted alphabetically by the first character of a given string of words.
Sample Data:
(“Red Green Black White Pink”) -> “Black Green Pink Red White”
(“Calculate the sum of two said numbers given as strings.”) -> (“Calculate as given numbers of sum said strings. the two”)
("The quick brown fox jumps over the lazy dog.") -> (“The brown dog. fox jumps lazy over quick the”)
Sample Solution-1:
Python Code:
# Define a function that sorts words in a string based on their first character
def test(text):
# Split the input string into words, sort them based on the first character, and join them back into a string
return ' '.join(sorted(text.split(), key=lambda c: c[0]))
# Initialize a string with words
word = "Red Green Black White Pink"
# Print the original word
print("Original Word:", word)
# Print a message indicating sorting based on the first character
print("Sort the said string based on its first character:")
# Call the function to sort the words and print the result
print(test(word))
# Repeat the process with different strings
word = "Calculate the sum of two said numbers given as strings."
print("\nOriginal Word:", word)
print("Sort the said string based on its first character:")
print(test(word))
word = "The quick brown fox jumps over the lazy dog."
print("\nOriginal Word:", word)
print("Sort the said string based on its first character:")
print(test(word))
Sample Output:
Original Word: Red Green Black White Pink Sort the said string based on its first character: Black Green Pink Red White Original Word: Calculate the sum of two said numbers given as strings. Sort the said string based on its first character: Calculate as given numbers of sum said strings. the two Original Word: The quick brown fox jumps over the lazy dog. Sort the said string based on its first character: The brown dog. fox jumps lazy over quick the
Flowchart:
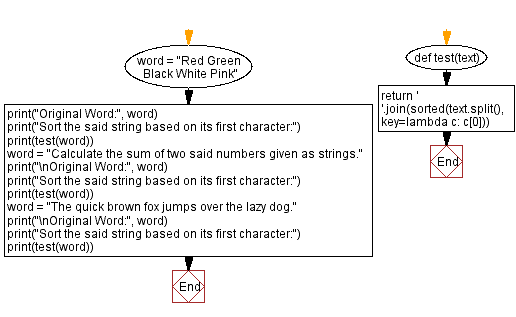
Sample Solution-2:
Python Code:
# Define a function that sorts words in a string based on their first character
def test(text):
# Split the input string into a list of words
data = text.split(' ')
# Initialize an empty list to store the sorted words
result = []
# Extract the first character of each word and sort them
char = sorted([i[0] for i in data])
# Iterate over the sorted characters
for i in char:
# Iterate over the original words
for j in data:
# Check if the first character of the word matches the current sorted character
# and if the word is not already in the result list
if i == j[0] and j not in result:
# Append the word to the result list
result.append(j)
# Join the sorted words into a string and return
return ' '.join(result)
# Initialize a string with words
word = "Red Green Black White Pink"
# Print the original word
print("Original Word:", word)
# Print a message indicating sorting based on the first character
print("Sort the said string based on its first character:")
# Call the function to sort the words and print the result
print(test(word))
# Repeat the process with different strings
word = "Calculate the sum of two said numbers given as strings."
print("\nOriginal Word:", word)
print("Sort the said string based on its first character:")
print(test(word))
word = "The quick brown fox jumps over the lazy dog."
print("\nOriginal Word:", word)
print("Sort the said string based on its first character:")
print(test(word))
Sample Output:
Original Word: Red Green Black White Pink Sort the said string based on its first character: Black Green Pink Red White Original Word: Calculate the sum of two said numbers given as strings. Sort the said string based on its first character: Calculate as given numbers of sum said strings. the two Original Word: The quick brown fox jumps over the lazy dog. Sort the said string based on its first character: The brown dog. fox jumps lazy over quick the
Flowchart:
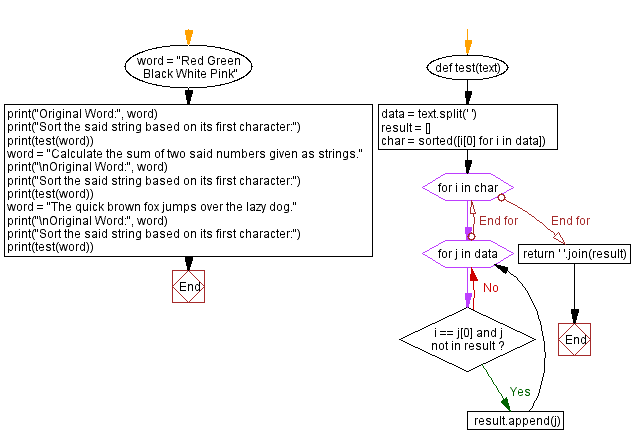
Python Code Editor:
Previous Python Exercise: Calculate the sum of two numbers given as strings.
Next Python Exercise: Python List Exercise Home.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-113.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics