Python: Count the occurrences of each word in a given sentence
Count word occurrences in a sentence.
Write a Python program to count the occurrences of each word in a given sentence.
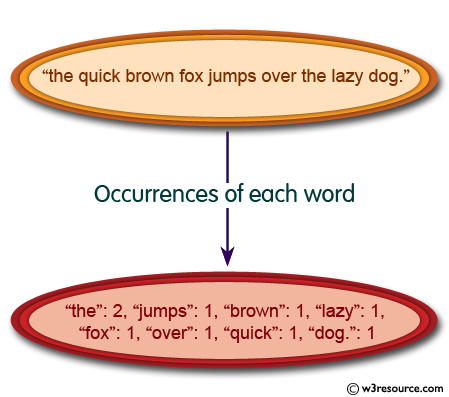
Sample Solution:
Python Code:
# Define a function named word_count that takes one argument, 'str'.
def word_count(str):
# Create an empty dictionary named 'counts' to store word frequencies.
counts = dict()
# Split the input string 'str' into a list of words using spaces as separators and store it in the 'words' list.
words = str.split()
# Iterate through each word in the 'words' list.
for word in words:
# Check if the word is already in the 'counts' dictionary.
if word in counts:
# If the word is already in the dictionary, increment its frequency by 1.
counts[word] += 1
else:
# If the word is not in the dictionary, add it to the dictionary with a frequency of 1.
counts[word] = 1
# Return the 'counts' dictionary, which contains word frequencies.
return counts
# Call the word_count function with an input sentence and print the results.
print( word_count('the quick brown fox jumps over the lazy dog.'))
Sample Output:
{'the': 2, 'jumps': 1, 'brown': 1, 'lazy': 1, 'fox': 1, 'over': 1, 'quick': 1, 'dog.': 1}
Flowchart:
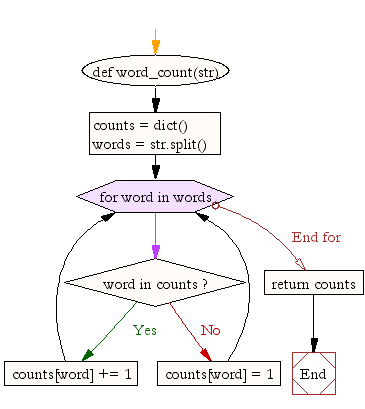
For more Practice: Solve these Related Problems:
- Write a Python program to count the frequency of each word in a sentence and return a dictionary of word counts.
- Write a Python program to split a sentence into words and then use a loop to build a frequency table.
- Write a Python program to implement word counting using collections.Counter on the split sentence.
- Write a Python program to count word occurrences while ignoring case and punctuation.
Go to:
Previous: Write a Python program to remove the characters which have odd index values of a given string.
Next: Write a Python script that takes input from the user and displays that input back in upper and lower cases.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.