Python: Prints the unique words in sorted form from a comma separated sequence of words
Sort distinct words in comma-separated input.
Write a Python program that accepts a comma-separated sequence of words as input and prints the distinct words in sorted form (alphanumerically).
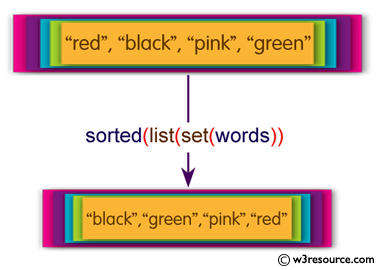
Sample Solution:
Python Code:
# Prompt the user to input a comma-separated sequence of words and store it in the variable 'items'.
items = input("Input comma-separated sequence of words")
# Split the input 'items' into a list of words by using the comma as the separator and store it in the 'words' list.
words = [word for word in items.split(",")]
# Convert the 'words' list into a set to remove any duplicate words, then convert it back to a list.
# Sort the resulting list alphabetically and join the words with commas.
# Finally, print the sorted and comma-separated list of unique words.
print(",".join(sorted(list(set(words)))))
Sample Output:
Input comma separated sequence of words red, black, pink, green black, green, pink, red
Flowchart:
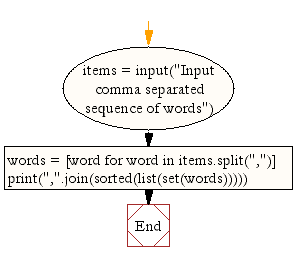
For more Practice: Solve these Related Problems:
- Write a Python program to accept a comma-separated sequence of words, remove duplicates, and output the distinct words in sorted order.
- Write a Python program to process a string of comma-separated words and print the unique words alphabetically.
- Write a Python program to split the input string on commas, trim spaces, and sort the distinct words.
- Write a Python program to use set() and sorted() to extract and order unique words from comma-separated input.
Go to:
Previous: Write a Python script that takes input from the user and displays that input back in upper and lower cases.
Next: Write a Python function to create the HTML string with tags around the word(s).
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.