Python: Create the HTML string with tags around the word(s)
Wrap word(s) in HTML tags.
Write a Python function to create an HTML string with tags around the word(s).
Sample function and result :
add_tags('i', 'Python') -> '<i>Python</i>'
add_tags('b', 'Python Tutorial') -> '<b>Python Tutorial </b>'
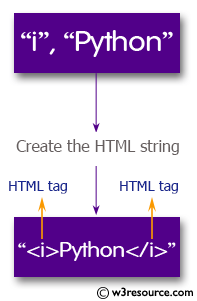
Sample Solution:
Python Code:
# Define a function named add_tags that takes two arguments, 'tag' and 'word'.
def add_tags(tag, word):
# Use string formatting to create and return a new string with the provided 'tag' enclosing the 'word'.
return "<%s>%s</%s>" % (tag, word, tag)
# Call the add_tags function with the tag 'i' and the word 'Python' and print the result.
print(add_tags('i', 'Python'))
# Call the add_tags function with the tag 'b' and the word 'Python Tutorial' and print the result.
print(add_tags('b', 'Python Tutorial'))
Sample Output:
<i>Python</i> <b>Python Tutorial</b>
Flowchart:
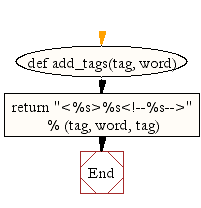
For more Practice: Solve these Related Problems:
- Write a Python function to enclose a given word with specified tags using string concatenation.
- Write a Python program to generate an element by wrapping input text with provided tag names.
- Write a Python function that takes a tag and a string, then returns the string enclosed within opening and closing tags.
- Write a Python program to implement a decorator that wraps function output in tags.
Go to:
Previous: Write a Python program that accepts a comma separated sequence of words as input and prints the unique words in sorted form (alphanumerically).
Next: Write a Python function to insert a string in the middle of a string.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.