Python: Get a string made of 4 copies of the last two characters of a specified string
Python String: Exercise-17 with Solution
Write a Python function to get a string made of 4 copies of the last two characters of a specified string (length must be at least 2).
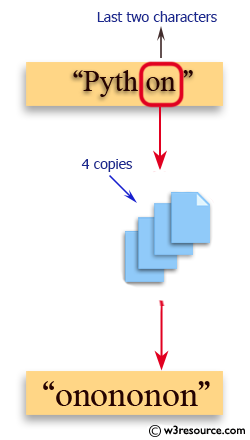
Sample Solution:
Python Code:
# Define a function named insert_end that takes one argument, 'str'.
def insert_end(str):
# Extract the last two characters from the input string 'str' and store them in 'sub_str'.
sub_str = str[-2:]
# Multiply 'sub_str' by 4 and return the result, effectively repeating the last two characters four times.
return sub_str * 4
# Call the insert_end function with different input strings and print the results.
print(insert_end('Python')) # Output: 'onononon'
print(insert_end('Exercises')) # Output: 'eseseses'
Sample Output:
onononon eseseses
Flowchart:
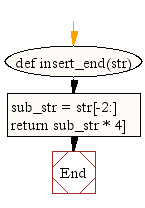
Python Code Editor:
Previous: Write a Python function to insert a string in the middle of a string.
Next: Write a Python function to get a string made of its first three characters of a specified string. If the length of the string is less than 3 then return the original string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-17.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics