Python: Get the last part of a string before a specified character
Get substring before a specific character.
Write a Python program to get the last part of a string before a specified character.
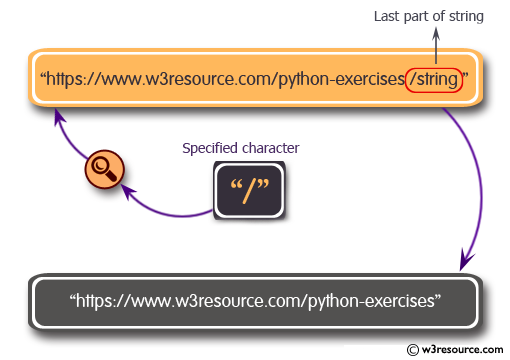
Sample Solution:
Python Code:
# Define a variable 'str1' and assign it the value of the provided string.
str1 = 'https://www.w3resource.com/python-exercises/string'
# Use the rsplit() method with '/' as the separator to split the string from the right,
# and [0] to get the part before the last '/' character. Then, print the result.
print(str1.rsplit('/', 1)[0]) # Output: 'https://www.w3resource.com/python-exercises'
# Use the rsplit() method with '-' as the separator to split the string from the right,
# and [0] to get the part before the last '-' character. Then, print the result.
print(str1.rsplit('-', 1)[0]) # Output: 'https://www.w3resource.com/python'
Sample Output:
https://www.w3resource.com/python-exercises https://www.w3resource.com/python
Flowchart:
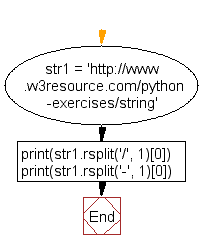
For more Practice: Solve these Related Problems:
- Write a Python program to split a string at the first occurrence of a specified character and return the substring before it.
- Write a Python program to use the partition() method to extract the part of a string before a given delimiter.
- Write a Python program to iterate over a string and build the substring until a specified character is encountered.
- Write a Python program to implement this logic recursively, returning the substring before the target character.
Go to:
Previous: Write a Python function to get a string made of its first three characters of a specified string. If the length of the string is less than 3 then return the original string.
Next: Write a Python function to reverse a string if it's length is a multiple of 4.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.