Python: Reverse a string if it's length is a multiple of 4
Python String: Exercise-20 with Solution
Write a Python function to reverse a string if its length is a multiple of 4.
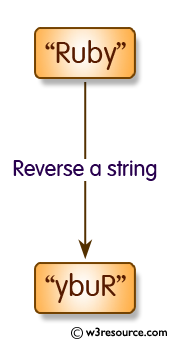
Sample Solution:
Python Code:
# Define a function named reverse_string that takes one argument, 'str1'.
def reverse_string(str1):
# Check if the length of the input string 'str1' is divisible by 4 with no remainder.
if len(str1) % 4 == 0:
# If the length is divisible by 4, reverse the characters in 'str1' and join them together.
return ''.join(reversed(str1))
# If the length of 'str1' is not divisible by 4, return 'str1' as it is.
return str1
# Call the reverse_string function with different input strings and print the results.
print(reverse_string('abcd')) # Output: 'dcba' (Reversed)
print(reverse_string('python')) # Output: 'python' (Not reversed)
Sample Output:
dcba python
Flowchart:
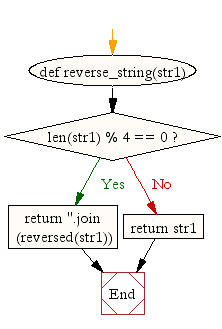
Python Code Editor:
Previous: Write a Python program to get the last part of a string before a specified character.
Next: Write a Python function to convert a given string to all uppercase if it contains at least 2 uppercase characters in the first 4 characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-20.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics