Python: Convert a string to all uppercase if it contains at least 2 uppercase characters in the first 4 characters
Python String: Exercise-21 with Solution
Write a Python function to convert a given string to all uppercase if it contains at least 2 uppercase characters in the first 4 characters.
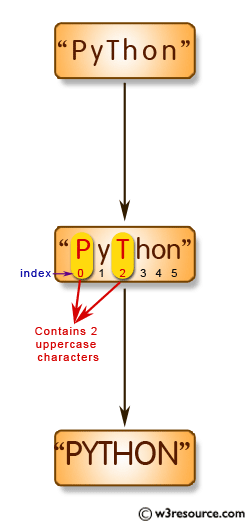
Sample Solution:
Python Code:
# Define a function named to_uppercase that takes one argument, 'str1'.
def to_uppercase(str1):
# Initialize a variable 'num_upper' to count the uppercase letters in the first 4 characters of 'str1'.
num_upper = 0
# Iterate through the first 4 characters of 'str1'.
for letter in str1[:4]:
# Check if the uppercase version of the letter is the same as the original letter.
if letter.upper() == letter:
# If they are the same, increment the 'num_upper' count.
num_upper += 1
# Check if the count of uppercase letters is greater than or equal to 2.
if num_upper >= 2:
# If there are 2 or more uppercase letters in the first 4 characters, return 'str1' in all uppercase.
return str1.upper()
# If there are fewer than 2 uppercase letters in the first 4 characters, return 'str1' as it is.
return str1
# Call the to_uppercase function with different input strings and print the results.
print(to_uppercase('Python')) # Output: 'Python' (Not all uppercase)
print(to_uppercase('PyThon')) # Output: 'PYTHON' (All uppercase)
Sample Output:
Python PYTHON
Flowchart:
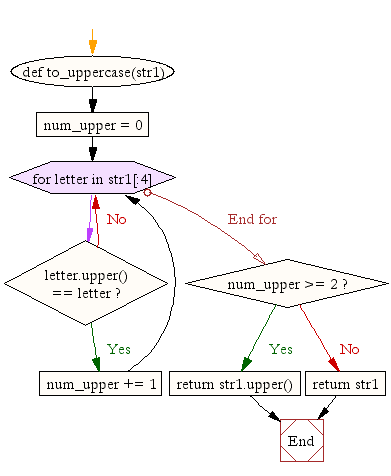
Python Code Editor:
Previous: Write a Python function to reverse a string if it's length is a multiple of 4.
Next: Write a Python program to sort a string lexicographically.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-21.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics