Python: Reverse a string
Reverse a string.
Write a Python program to reverse a string.
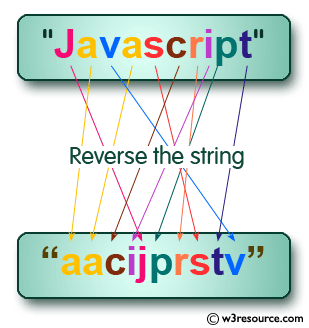
Sample Solution:
Python Code:
# Define a function 'reverse_string' that takes a string 'str1' as input.
# The function returns the reversed version of the input string.
def reverse_string(str1):
return ''.join(reversed(str1))
# Print an empty line for spacing.
print()
# Call the 'reverse_string' function with the input "abcdef", reverse the string, and print the result.
print(reverse_string("abcdef"))
# Call the 'reverse_string' function with the input "Python Exercises.", reverse the string, and print the result.
print(reverse_string("Python Exercises."))
# Print an empty line for spacing.
print()
Sample Output:
fedcba .sesicrexE nohtyP
Flowchart:
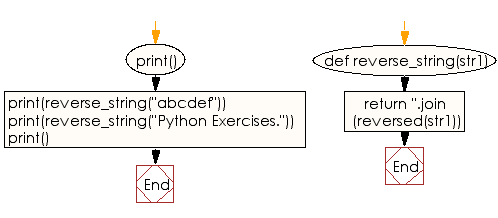
For more Practice: Solve these Related Problems:
- Write a Python program to reverse a string using slicing.
- Write a Python program to reverse a string using a for-loop and concatenation.
- Write a Python program to convert a string to a list, reverse the list, and then join it back into a string.
- Write a Python program to implement a recursive function that reverses a string character by character.
Go to:
Previous: Write a Python program to count occurrences of a substring in a string.
Next: Write a Python program to reverse words in a string.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.