Python: Reverse words in a string
Python String: Exercise-40 with Solution
Write a Python program to reverse words in a string.
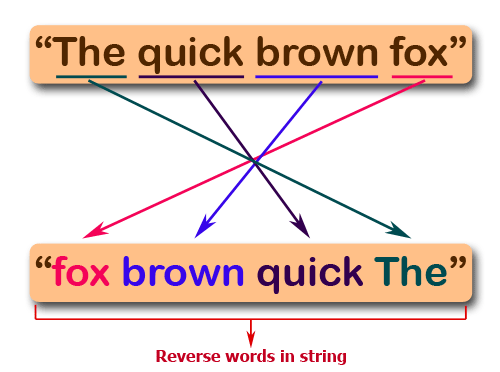
Sample Solution:
Python Code:
# Define a function 'reverse_string_words' that takes a string 'text' as input.
# The function splits the input text into lines, reverses the words within each line, and returns the result.
def reverse_string_words(text):
for line in text.split('\n'):
return(' '.join(line.split()[::-1]))
# Call the 'reverse_string_words' function with the input "The quick brown fox jumps over the lazy dog.",
# reverse the words in the string, and print the result.
print(reverse_string_words("The quick brown fox jumps over the lazy dog."))
# Call the 'reverse_string_words' function with the input "Python Exercises.",
# reverse the words in the string, and print the result.
print(reverse_string_words("Python Exercises."))
Sample Output:
dog. lazy the over jumps fox brown quick The Exercises. Python
Flowchart:
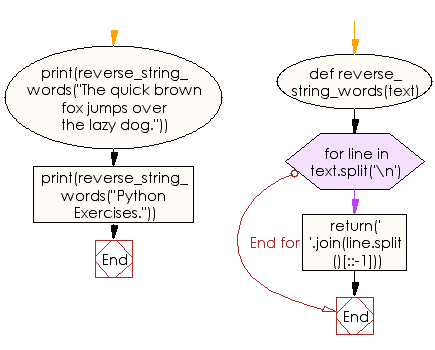
Python Code Editor:
Previous: Write a Python program to reverse a string.
Next: Write a Python program to strip a set of characters from a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-40.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics