Python: Count and display the vowels of a given text
Python String: Exercise-49 with Solution
Write a Python program to count and display vowels in text.
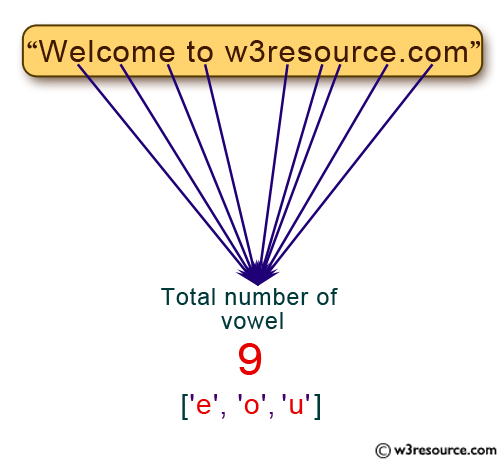
Sample Solution:
Python Code:
# Define a function 'vowel' that takes a string 'text' as input.
def vowel(text):
# Define a string 'vowels' containing all lowercase and uppercase vowels.
vowels = "aeiuoAEIOU"
# Print the number of vowels in the input string 'text' using a list comprehension.
print(len([letter for letter in text if letter in vowels]))
# Print a list of vowels found in the input string 'text' using a list comprehension.
print([letter for letter in text if letter in vowels])
# Call the 'vowel' function with the input string 'w3resource'.
vowel('w3resource')
Sample Output:
4 ['e', 'o', 'u', 'e']
Flowchart:
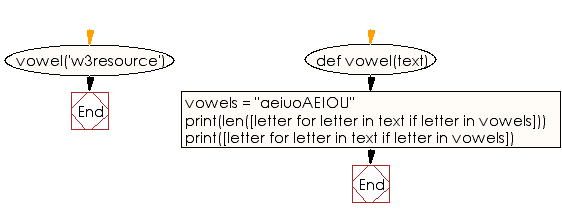
Python Code Editor:
Previous: Write a Python program to swap comma and dot in a string.
Next: Write a Python program to split a string on the last occurrence of the delimiter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-49.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics