Python: Find the first repeated word in a given string
Python String: Exercise-55 with Solution
Write a Python program to find the first repeated word in a given string.
Visual Presentation:
Sample Solution:
Python Code:
# Define a function 'first_repeated_word' that takes a string 'str1' as input.
def first_repeated_word(str1):
# Create an empty set 'temp' to store unique words encountered.
temp = set()
# Iterate through each word in the string 'str1' by splitting it using spaces.
for word in str1.split():
# Check if the word is already in the 'temp' set.
if word in temp:
return word # Return the first repeated word found.
else:
temp.add(word) # Add the word to the 'temp' set if it's not already there.
return 'None' # Return 'None' if no repeated word is found.
# Call the 'first_repeated_word' function with different input strings and print the results.
print(first_repeated_word("ab ca bc ab"))
print(first_repeated_word("ab ca bc ab ca ab bc"))
print(first_repeated_word("ab ca bc ca ab bc"))
print(first_repeated_word("ab ca bc"))
Sample Output:
ab ab ca None
Flowchart:
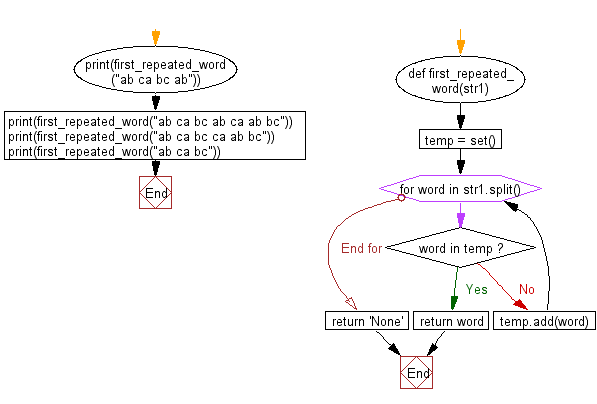
Python Code Editor:
Previous: Write a Python program to find the first repeated character of a given string where the index of first occurrence is smallest.
Next:Write a Python program to find the second most repeated word in a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-55.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics