Python: Find the maximum occurring character in a given string
Find maximum occurring character.
Write a Python program to find the maximum number of characters in a given string.
Visual Presentation:
Sample Solution:
Python Code:
# Define a function 'get_max_occuring_char' that takes a string 'str1' as input.
def get_max_occuring_char(str1):
# Define the size of the ASCII character set (256 characters).
ASCII_SIZE = 256
# Create a list 'ctr' with 256 elements, initialized to 0. It will store the count of each character.
ctr = [0] * ASCII_SIZE
# Initialize variables 'max' to -1 and 'ch' to an empty string.
max = -1
ch = ''
# Iterate through each character 'i' in the input string 'str1'.
for i in str1:
# Increment the count of the character 'i' in the 'ctr' list.
ctr[ord(i)] += 1
# Iterate through each character 'i' in the input string 'str1' again.
for i in str1:
# Check if the count of the character 'i' is greater than the current 'max'.
if max < ctr[ord(i)]:
max = ctr[ord(i)] # Update 'max' to the new maximum count.
ch = i # Update 'ch' to the character with the maximum count.
return ch # Return the character with the highest count.
# Call the 'get_max_occuring_char' function with different input strings and print the results.
print(get_max_occuring_char("Python: Get file creation and modification date/times"))
print(get_max_occuring_char("abcdefghijkb"))
Sample Output:
t b
Flowchart:
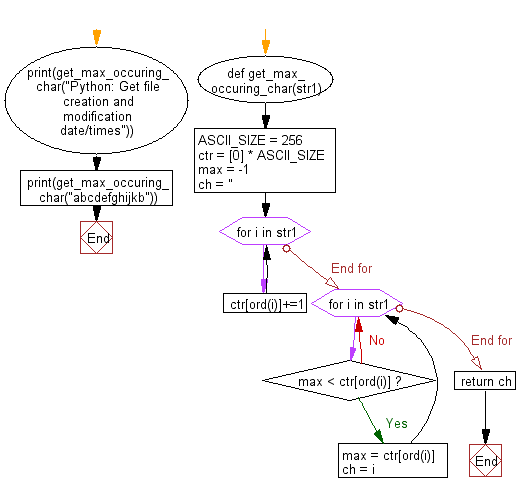
For more Practice: Solve these Related Problems:
- Write a Python program to count character frequencies in a string and return the character with the highest count.
- Write a Python program to use collections.Counter to find the most common character in a string.
- Write a Python program to iterate through a string and identify the character that appears the most, handling ties by lexicographical order.
- Write a Python program to implement a function that returns both the maximum occurring character and its frequency.
Go to:
Previous: Write a Python program to move spaces to the front of a given string.
Next: Write a Python program to capitalize first and last letters of each word of a given string.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.