Python: Compute sum of digits of a given string
Python String: Exercise-62 with Solution
Write a Python program to compute the sum of the digits in a given string.
Visual Presentation:
Sample Solution:
Python Code:
# Define a function to calculate the sum of digits in a string
def sum_digits_string(str1):
# Initialize a variable to store the sum of digits
sum_digit = 0
# Iterate over each character in the string
for char in str1:
# Check if the current character is a digit
if char.isdigit():
# Convert the digit character to an integer
digit = int(char)
# Add the digit to the sum
sum_digit += digit
# Return the sum of digits
return sum_digit
# Calculate the sum of digits in the string "123abcd45"
result1 = sum_digits_string("123abcd45")
print(result1) # Output: 15
# Calculate the sum of digits in the string "abcd1234"
result2 = sum_digits_string("abcd1234")
print(result2) # Output: 10
Sample Output:
15 10
Flowchart:
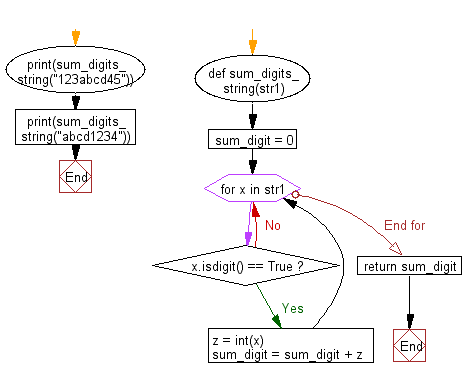
Python Code Editor:
Previous: Write a Python program to remove duplicate characters of a given string.
Next: Write a Python program to remove leading zeros from an IP address.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-62.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics