Python: Remove leading zeros from an IP address
Remove leading zeros in IP address.
Write a Python program to remove leading zeros from an IP address.
Pictorial Presentation:
Sample Solution:
Python Code:
# Define a function that takes an IP address as input and removes leading zeros from each octet
def remove_zeros_from_ip(ip_add):
# Split the IP address into its octets and convert each octet to an integer with no leading zeros
new_ip_add = ".".join([str(int(i)) for i in ip_add.split(".")])
# Return the new IP address with no leading zeros
return new_ip_add ;
# Test the function with two example IP addresses
print(remove_zeros_from_ip("255.024.01.01"))
print(remove_zeros_from_ip("127.0.0.01 "))
Sample Output:
255.24.1.1 127.0.0.1
Flowchart:
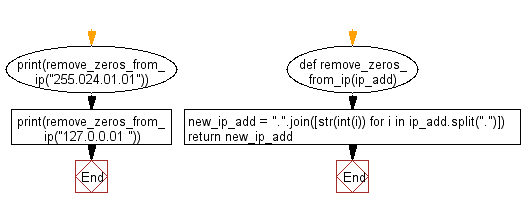
Python Code Editor:
Previous: Write a Python program to compute sum of digits of a given string.
Next: Write a Python program to find maximum length of consecutive 0’s in a given binary string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics