Python: Find all the common characters in lexicographical order from two given lower case strings
Common characters between two strings.
Write a Python program to find all the common characters in lexicographical order from two given lower case strings. If there are no similar letters print "No common characters".
Visual Presentation:
Sample Solution:
Python Code:
# Import Counter for counting characters
from collections import Counter
def common_chars(str1,str2):
# Create Counter objects for each string
d1 = Counter(str1)
d2 = Counter(str2)
# Intersection of the counters gives common elements
common_dict = d1 & d2
# If no common elements, return message
if len(common_dict) == 0:
return "No common characters."
# Get list of common characters
common_chars = list(common_dict.elements())
# Sort common characters
common_chars = sorted(common_chars)
# Join the characters into a string
return ''.join(common_chars)
# Test strings
str1 = 'Python'
str2 = 'PHP'
# Print test strings
print("Two strings: "+str1+' : '+str2)
# Print common characters
print(common_chars(str1, str2))
# Test with different strings
str1 = 'Java'
str2 = 'PHP'
# Print test strings
print("Two strings: "+str1+' : '+str2)
# Print common characters
print(common_chars(str1, str2))
Sample Output:
Two strings: Python : PHP P Two strings: Java : PHP No common characters.
Flowchart:
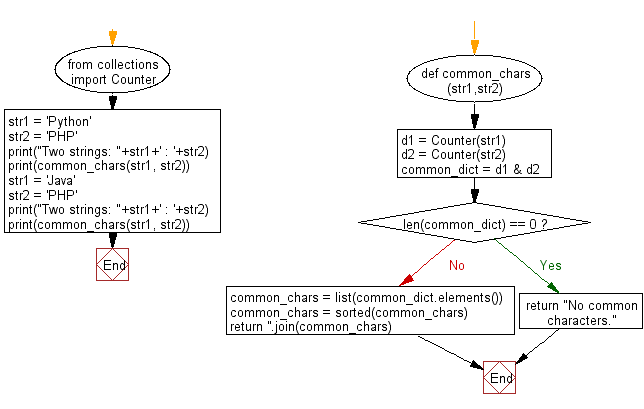
Python Code Editor:
Previous: Write a Python program to find maximum length of consecutive 0’s in a given binary string.
Next: Write a Python program to make two given strings (lower case, may or may not be of the same length) anagrams removing any characters from any of the strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics