Python: Create two strings from a given string
Python String: Exercise-68 with Solution
Write a Python program to generate two strings from a given string. For the first string, use the characters that occur only once, and for the second, use the characters that occur multiple times in the said string.
Visual Presentation:
Sample Solution:
Python Code:
# Import Counter from collections
from collections import Counter
# Function to generate two strings
def generateStrings(input):
# Create a character counter from input
str_char_ctr = Counter(input)
# Part 1 contains single occurrence characters
part1 = [key for (key,count) in str_char_ctr.items() if count==1]
# Part 2 contains multiple occurrence characters
part2 = [key for (key,count) in str_char_ctr.items() if count>1]
# Sort the characters in each part
part1.sort()
part2.sort()
return part1,part2
# Test input string
input = "aabbcceffgh"
# Generate the two strings
s1, s2 = generateStrings(input)
# Print first string
print(''.join(s1))
# Print second string
print(''.join(s2))
Sample Output:
egh abcf
Flowchart:
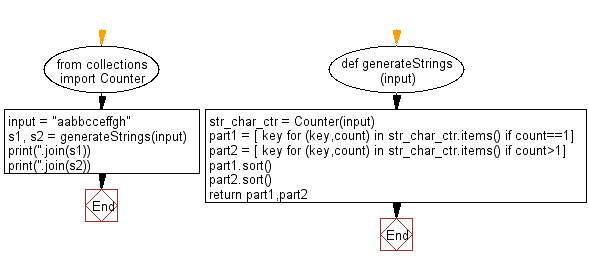
Python Code Editor:
Previous: Write a Python program to remove all consecutive duplicates of a given string.
Next: Write a Python program to find the longest common sub-string from two given strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-68.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics