Python: Find the longest common sub-string from two given strings
Python String: Exercise-69 with Solution
Write a Python program to find the longest common sub-string from two given strings.
Visual Presentation:
Sample Solution:
Python Code:
# Import SequenceMatcher from difflib
from difflib import SequenceMatcher
# Function to find longest common substring
def longest_Substring(s1,s2):
# Create sequence matcher object
seq_match = SequenceMatcher(None,s1,s2)
# Find longest matching substring
match = seq_match.find_longest_match(0, len(s1), 0, len(s2))
# If match found, return substring
if (match.size!=0):
return (s1[match.a: match.a + match.size])
# Else no match found
else:
return ('Longest common sub-string not present')
# Test strings
s1 = 'abcdefgh'
s2 = 'xswerabcdwd'
# Print original strings
print("Original Substrings:\n",s1+"\n",s2)
# Print message
print("\nCommon longest sub_string:")
# Print longest common substring
print(longest_Substring(s1,s2))
Sample Output:
Original Substrings: abcdefgh xswerabcdwd Common longest sub_string: abcd Original Substrings: abcdefgh xswerabcdwd Common longest sub_string: abcd
Flowchart:
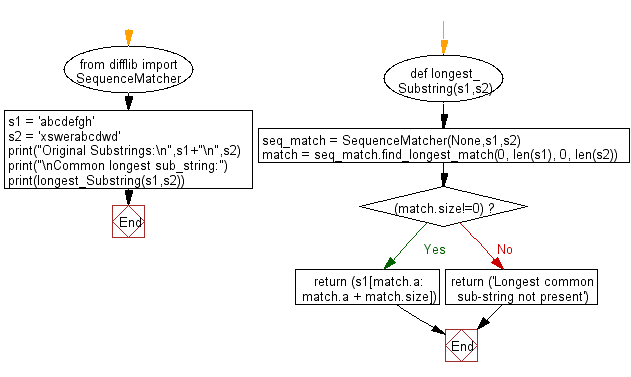
Python Code Editor:
Previous: Write a Python program to create two strings from a given string. Create the first string using those character which occurs only once and create the second string which consists of multi-time occurring characters in the said string.
Next: Write a Python program to create a string from two given strings concatenating uncommon characters of the said strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-69.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics