Python: Move all spaces to the front of a given string in single traversal
Python String: Exercise-71 with Solution
Write a Python program to move all spaces to the front of a given string in a single traversal.
Visual Presentation:
Sample Solution:
Python Code:
# Function to move spaces to front
def moveSpaces(str1):
# List comprehension to remove spaces
no_spaces = [char for char in str1 if char!=' ']
# Get number of spaces
space = len(str1) - len(no_spaces)
# Create string with required spaces
result = ' '*space
# Return moved spaces + string without spaces
return result + ''.join(no_spaces)
# Test string
s1 = "Python Exercises"
# Print original string
print("Original String:\n",s1)
# Print message
print("\nAfter moving all spaces to the front:")
# Print result
print(moveSpaces(s1))
Sample Output:
Original String: Python Exercises After moving all spaces to the front: PythonExercises
Flowchart:
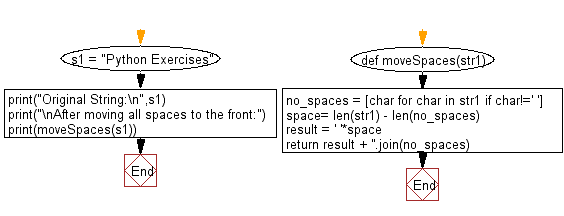
Python Code Editor:
Previous: Write a Python program to create a string from two given strings concatenating uncommon characters of the said strings.
Next: Write a Python code to remove all characters except a specified character in a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-71.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics