Python: Remove all characters except a specified character in a given string
Remove all except specified character.
Write a Python program to remove all characters except a specified character from a given string.
Sample Solution:
Python Code:
# Function to remove all chars except given char
def remove_characters(str1,c):
# List comprehension to keep only given char
return ''.join([el for el in str1 if el == c])
# Test string
text = "Python Exercises"
# Print original string
print("Original string")
print(text)
# Character to keep
except_char = "P"
# Print message and result
print("Remove all characters except",except_char,"in the said string:")
print(remove_characters(text,except_char))
# Second test string
text = "google"
print("\nOriginal string")
print(text)
except_char = "g"
# Print message and result
print("Remove all characters except",except_char,"in the said string:")
print(remove_characters(text,except_char))
# Third test string
text = "exercises"
print("\nOriginal string")
print(text)
except_char = "e"
# Print message and result
print("Remove all characters except",except_char,"in the said string:")
print(remove_characters(text,except_char))
Sample Output:
Original string Python Exercises Remove all characters except P in the said string: P Original string google Remove all characters except g in the said string: gg Original string exercises Remove all characters except e in the said string: eee
Flowchart:
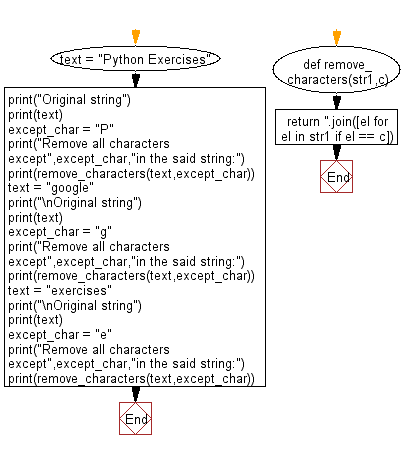
For more Practice: Solve these Related Problems:
- Write a Python program to remove all characters from a string except for a given target character.
- Write a Python program to filter a string and return only occurrences of a specified character.
- Write a Python program to implement a function that scans a string and constructs a new string consisting solely of the given character.
- Write a Python program to use list comprehension to retain only the specified character from the input string.
Go to:
Previous: Write a Python program to move all spaces to the front of a given string in single traversal.
Next: Write a Python program to count Uppercase, Lowercase, special character and numeric values in a given string.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.