Python: Count Uppercase, Lowercase, special character and numeric values in a given string
Python String: Exercise-73 with Solution
Write a Python program to count Uppercase, Lowercase, special characters and numeric values in a given string.
Visual Presentation:
Sample Solution:
Python Code:
# Function to count character types
def count_chars(str):
# Initialize counters
upper_ctr, lower_ctr, number_ctr, special_ctr = 0, 0, 0, 0
# Iterate through string
for i in range(len(str)):
# Increment appropriate counter
if str[i] >= 'A' and str[i] <= 'Z':
upper_ctr += 1
elif str[i] >= 'a' and str[i] <= 'z':
lower_ctr += 1
elif str[i] >= '0' and str[i] <= '9':
number_ctr += 1
else:
special_ctr += 1
# Return all counters
return upper_ctr, lower_ctr, number_ctr, special_ctr
# Test string
str = "@W3Resource.Com"
# Print original string
print("Original Substrings:",str)
# Call function and unpack counters
u, l, n, s = count_chars(str)
# Print counters
print('\nUpper case characters: ',u)
print('Lower case characters: ',l)
print('Number case: ',n)
print('Special case characters: ',s)
Sample Output:
Original Substrings: @W3Resource.Com Upper case characters: 3 Lower case characters: 9 Number case: 1 Special case characters: 2
Flowchart:
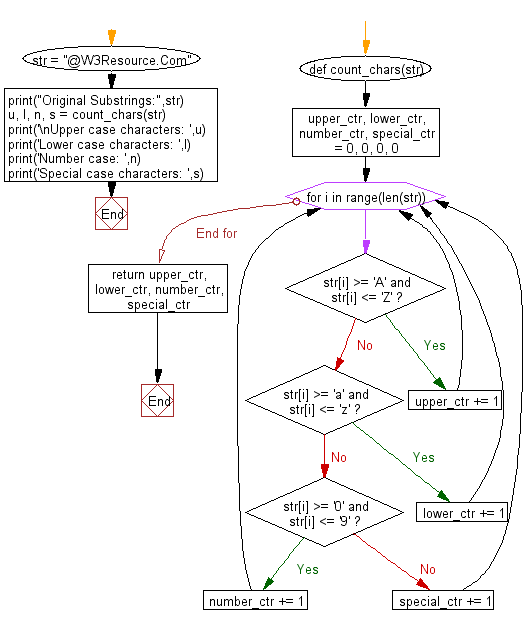
Python Code Editor:
Previous: Write a Python code to remove all characters except a specified character in a given string.
Next: Write a Python program to find the minimum window in a given string which will contain all the characters of another given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-73.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics