Python: Count number of substrings with same first and last characters of a given string
Substrings with same first and last char.
Write a Python program to count the number of substrings with the same first and last characters in a given string.
Visual Presentation:
Sample Solution:
Python Code:
# Function to count substrings with equal ends
def no_of_substring_with_equalEnds(str1):
result = 0;
# Get string length
n = len(str1);
# Generate all substrings
for i in range(n):
for j in range(i, n):
# Check if ends are equal
if (str1[i] == str1[j]):
result = result + 1
return result
# Get input string
str1 = input("Input a string: ")
# Print result
print(no_of_substring_with_equalEnds(str1))
Sample Output:
Input a string: abc 3
Flowchart:
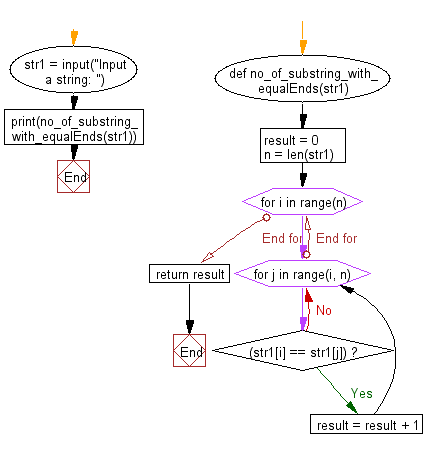
For more Practice: Solve these Related Problems:
- Write a Python program to count the number of substrings in a string where the first and last characters are identical.
- Write a Python program to generate all substrings that begin and end with the same character and return their count.
- Write a Python program to use nested loops to check each substring for matching first and last characters and tally the total.
- Write a Python program to implement this substring check using recursion and compare the results with an iterative approach.
Go to:
Previous: Write a Python program to find smallest and largest word in a given string.
Next: Write a Python program to find the index of a given string at which a given substring starts. If the substring is not found in the given string return 'Not found'.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.