Python: Find the index of a given string at which a given substring starts
Python String: Exercise-81 with Solution
Write a Python program to determine the index of a given string at which a certain substring starts. If the substring is not found in the given string return 'Not found'.
Sample Solution:
Python Code:
# Function to find index of substring
def find_Index(str1, pos):
# Check if pos longer than str1
if len(pos) > len(str1):
return 'Not found'
# Iterate through str1
for i in range(len(str1)):
# Iterate through pos
for j in range(len(pos)):
# If match found, return index
if str1[i + j] == pos[j] and j == len(pos) - 1:
return i
# Break if mismatch
elif str1[i + j] != pos[j]:
break
return 'Not found'
# Test cases
print(find_Index("Python Exercises", "Ex"))
print(find_Index("Python Exercises", "yt"))
print(find_Index("Python Exercises", "PY"))
Sample Output:
7 1 Not found
Flowchart:
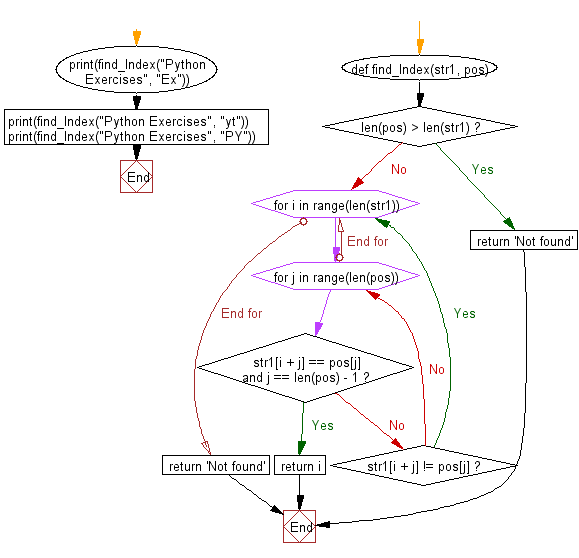
Python Code Editor:
Previous: Write a Python program to count number of substrings with same first and last characters of a given string.
Next: Write a Python program to wrap a given string into a paragraph of given width.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-81.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics