Python: Print four values decimal, octal, hexadecimal, binary in a single line of a given integer
Python String: Exercise-83 with Solution
Write a Python program to print four integer values - decimal, octal, hexadecimal (capitalized), binary - in a single line.
Sample Solution:
Python Code:
# Get user input for an integer
i = int(input("Input an integer: "))
# Convert the integer to octal and remove the '0o' prefix
o = str(oct(i))[2:]
# Convert the integer to hexadecimal and remove the '0x' prefix, then capitalize the result
h = str(hex(i))[2:].upper()
# Convert the integer to binary and remove the '0b' prefix
b = str(bin(i))[2:]
# Convert the integer to a string for decimal representation
d = str(i)
# Print the formatted output with headers
print("Decimal Octal Hexadecimal (capitalized), Binary")
print(d,' ',o,' ',h,' ',b)
Sample Output:
Input an integer: 25 Decimal Octal Hexadecimal (capitalized), Binary 25 31 19 11001
Flowchart:
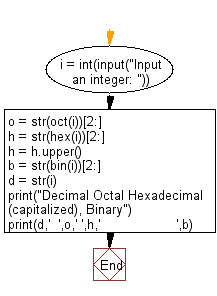
Python Code Editor:
Previous: Write a Python program to wrap a given string into a paragraph of given width.
Next: Write a Python program to swap cases of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-83.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics