Python: Swap cases of a given string
Swap cases in string.
Write a Python program to swap cases in a given string.
Sample Solution:
Python Code:
# Define a function to swap the case of characters in a string
def swap_case_string(str1):
# Initialize an empty string to store the result
result_str = ""
# Iterate through each character in the input string
for item in str1:
# Check if the character is uppercase
if item.isupper():
# If uppercase, convert to lowercase and append to the result string
result_str += item.lower()
else:
# If lowercase, convert to uppercase and append to the result string
result_str += item.upper()
# Return the final swapped case string
return result_str
# Test the function with different input strings and print the results
print(swap_case_string("Python Exercises"))
print(swap_case_string("Java"))
print(swap_case_string("NumPy"))
Sample Output:
pYTHON eXERCISES jAVA nUMpY
Flowchart:
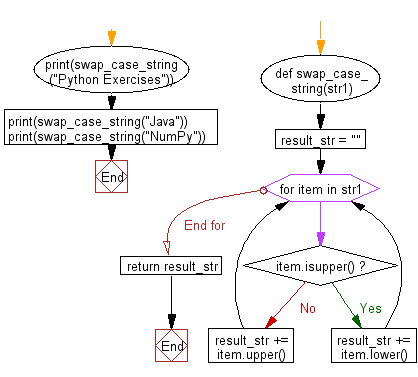
For more Practice: Solve these Related Problems:
- Write a Python program to swap the case of each letter in a string using the swapcase() method.
- Write a Python program to implement case swapping manually by iterating over each character and toggling its case.
- Write a Python program to use a list comprehension to produce a new string where uppercase letters become lowercase and vice versa.
- Write a Python program to combine slicing and conditional logic to swap cases in a given string without built-in methods.
Go to:
Previous: Write a Python program to print four values decimal, octal, hexadecimal (capitalized), binary in a single line of a given integer.
Next: Write a Python program to convert a given Bytearray to Hexadecimal string.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.