Python: Delete all occurrences of a specified character in a given string
Delete all occurrences of character.
Write a Python program to delete all occurrences of a specified character in a given string.
Sample Solution:
Python Code:
# Define a function to delete all occurrences of a specified character in a given string
def delete_all_occurrences(str1, ch):
# Use the replace() method to replace all occurrences of the specified character with an empty string
result = str1.replace(ch, "")
# Return the modified string
return result
# Initialize a string
str_text = "Delete all occurrences of a specified character in a given string"
# Print the original string
print("Original string:")
print(str_text)
# Print a newline for better formatting
print("\nModified string:")
# Specify the character to be deleted and call the function to delete all occurrences of that character
ch = 'a'
print(delete_all_occurrences(str_text, ch))
Sample Output:
Original string: Delete all occurrences of a specified character in a given string Modified string: Delete ll occurrences of specified chrcter in given string
Flowchart:
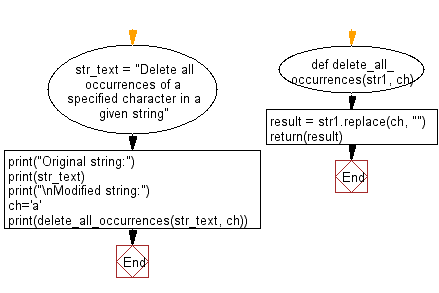
Python Code Editor:
Previous: Write a Python program to convert a given Bytearray to Hexadecimal string.
Next: Write a Python program find the common values that appear in two given strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics