Python: Find the common values that appear in two given strings
Python String: Exercise-87 with Solution
Write a Python program to find the common values that appear in two given strings.
Sample Solution:-
Python Code:
# Define a function to find the intersection of two strings
def intersection_of_two_string(str1, str2):
# Initialize an empty string to store the intersection
result = ""
# Iterate through each character in the first string
for ch in str1:
# Check if the character is present in the second string and not already in the result string
if ch in str2 and not ch in result:
# If true, add the character to the result string
result += ch
# Return the resulting intersection string
return result
# Initialize two strings
str1 = 'Python3'
str2 = 'Python2.7'
# Print the original strings
print("Original strings:")
print(str1)
print(str2)
# Print a newline for better formatting
print("\nIntersection of two said String:")
# Call the function to find the intersection of the two strings and print the result
print(intersection_of_two_string(str1, str2))
Sample Output:
Original strings: Python3 Python2.7 Intersection of two said String: Python
Flowchart:
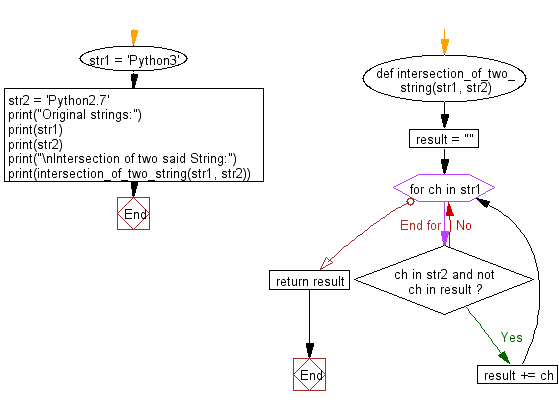
Python Code Editor:
Previous: Write a Python program to delete all occurrences of a specified character in a given string.
Next: Write a Python program to check whether a given string contains a capital letter, a lower case letter, a number and a minimum length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-87.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics