Python: Remove the nth index character from a nonempty string
Remove nth character from a string.
Write a Python program to remove the nth index character from a nonempty string.
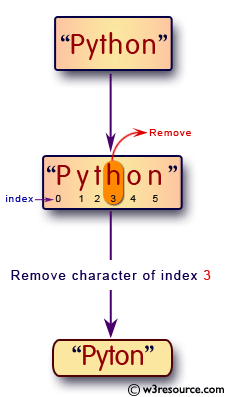
Sample Solution:
Python Code:
# Define a function named remove_char that takes two arguments, 'str' and 'n'.
def remove_char(str, n):
# Create a new string 'first_part' that includes all characters from the beginning of 'str' up to the character at index 'n' (not inclusive).
first_part = str[:n]
# Create a new string 'last_part' that includes all characters from the character at index 'n+1' to the end of 'str'.
last_part = str[n+1:]
# Return the result by concatenating 'first_part' and 'last_part', effectively removing the character at index 'n'.
return first_part + last_part
# Call the remove_char function with different input strings and character positions and print the results.
print(remove_char('Python', 0)) # Output: 'ython'
print(remove_char('Python', 3)) # Output: 'Pyton'
print(remove_char('Python', 5)) # Output: 'Pytho'
Sample Output:
ython Pyton Pytho
Flowchart:
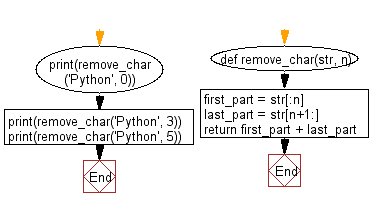
For more Practice: Solve these Related Problems:
- Write a Python program to remove the character at a specified index from a nonempty string using slicing.
- Write a Python program to implement a function that removes the nth character after validating the index.
- Write a Python program to create a new string by concatenating the parts before and after the nth character.
- Write a Python program to remove the nth character using recursion to reconstruct the string.
Go to:
Previous: Write a Python function that takes a list of words and return the longest word and the length of the longest one.
Next: Write a Python program to change a given string to a new string where the first and last chars have been exchanged.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.