Python: Remove duplicate words from a given string
Remove duplicate words in string.
Write a Python program to remove duplicate words from a given string.
Sample Solution:
Python Code:
# Define a function to return a string with unique words
def unique_list(text_str):
# Split the input string into a list of words
l = text_str.split()
# Initialize an empty list to store unique words
temp = []
# Iterate through each word in the list
for x in l:
# Check if the word is not already in the temporary list
if x not in temp:
# If true, add the word to the temporary list
temp.append(x)
# Join the unique words into a string and return the result
return ' '.join(temp)
# Initialize a string
text_str = "Python Exercises Practice Solution Exercises"
# Print the original string
print("Original String:")
print(text_str)
# Print a newline for better formatting
print("\nAfter removing duplicate words from the said string:")
# Call the function to remove duplicate words and print the result
print(unique_list(text_str))
Sample Output:
Original String: Python Exercises Practice Solution Exercises After removing duplicate words from the said string: Python Exercises Practice Solution
Flowchart:
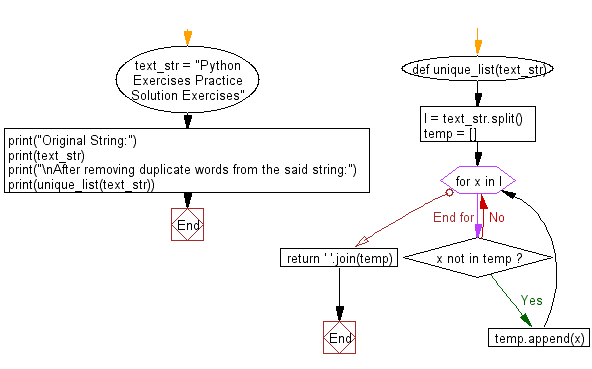
Python Code Editor:
Previous: Write a Python program to remove unwanted characters from a given string.
Next: Write a Python program to convert a given heterogeneous list of scalars into a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics