Python: Find the string similarity between two given strings
Python String: Exercise-92 with Solution
Write a Python program to find string similarity between two given strings.
From Wikipedia:
In computer science, approximate string matching (often colloquially referred to as fuzzy string searching) is the technique of finding strings that match a pattern approximately (rather than exactly).
Sample Solution:
Python Code:
# Import the difflib module for computing string similarity
import difflib
# Define a function to calculate the similarity ratio between two strings
def string_similarity(str1, str2):
# Create a SequenceMatcher object with the lowercase versions of the input strings
result = difflib.SequenceMatcher(a=str1.lower(), b=str2.lower())
# Return the similarity ratio between the two strings
return result.ratio()
# Initialize two strings for comparison
str1 = 'Python Exercises'
str2 = 'Python Exercises'
# Print the original strings
print("Original string:")
print(str1)
print(str2)
# Print a message indicating the similarity between the two strings
print("Similarity between two said strings:")
print(string_similarity(str1, str2))
# Repeat the process with different pairs of strings
str1 = 'Python Exercises'
str2 = 'Python Exercise'
print("\nOriginal string:")
print(str1)
print(str2)
print("Similarity between two said strings:")
print(string_similarity(str1, str2))
str1 = 'Python Exercises'
str2 = 'Python Ex.'
print("\nOriginal string:")
print(str1)
print(str2)
print("Similarity between two said strings:")
print(string_similarity(str1, str2))
str1 = 'Python Exercises'
str2 = 'Python'
print("\nOriginal string:")
print(str1)
print(str2)
print("Similarity between two said strings:")
print(string_similarity(str1, str2))
str1 = 'Python Exercises'
str2 = 'Java Exercises'
print("\nOriginal string:")
print(str1)
print(str2)
print("Similarity between two said strings:")
print(string_similarity(str1, str2))
Sample Output:
Original string: Python Exercises Python Exercises Similarity between two said strings: 1.0 Original string: Python Exercises Python Exercise Similarity between two said strings: 0.967741935483871 Original string: Python Exercises Python Ex. Similarity between two said strings: 0.6923076923076923 Original string: Python Exercises Python Similarity between two said strings: 0.5454545454545454 Original string: Java Exercises Python Similarity between two said strings: 0.0
Flowchart:
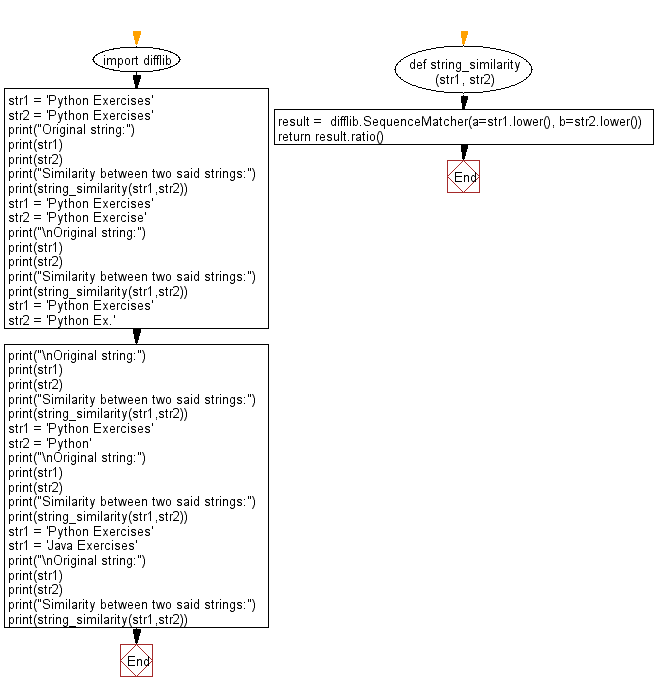
Python Code Editor:
Previous: Write a Python program to convert a given heterogeneous list of scalars into a string.
Next: Write a Python program to extract numbers from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-92.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics