Python: Extract numbers from a given string
Extract numbers from string.
Write a Python program to extract numbers from a given string.
Sample Solution:
Python Code:
# Define a function to extract numbers from a given string
def test(str1):
# Use a list comprehension to extract integers from the string if they are digits
result = [int(str1) for str1 in str1.split() if str1.isdigit()]
# Return the list of extracted numbers
return result
# Initialize a string containing a mix of words and numbers
str1 = "red 12 black 45 green"
# Print the original string
print("Original string:", str1)
# Print a message indicating the extraction of numbers from the string
print("Extract numbers from the said string:")
# Call the function to extract numbers and print the result
print(test(str1))
Sample Output:
Original string: red 12 black 45 green Extract numbers from the said string: [12, 45]
Flowchart:
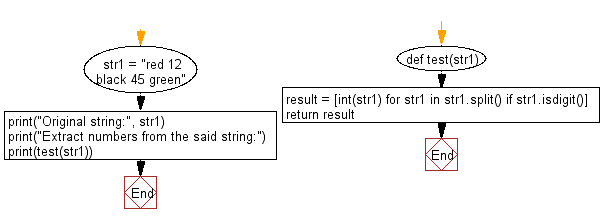
For more Practice: Solve these Related Problems:
- Write a Python program to extract all numeric substrings from a given string using regular expressions.
- Write a Python program to iterate over a string and return a list of all numbers found, converting them to integers.
- Write a Python program to use re.findall() to capture sequences of digits and output them as a list of integers.
- Write a Python program to implement a function that parses a string and returns all contiguous digit sequences as numbers.
Go to:
Previous: Write a Python program to find the string similarity between two given strings.
Next: Write a Python program to convert a hexadecimal color code to a tuple of integers corresponding to its RGB components.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.