Python: Convert a hexadecimal color code to its RGB components
Hex color code to RGB tuple.
Write a Python program to convert a hexadecimal color code to a tuple of integers corresponding to its RGB components.
- Use a list comprehension in combination with int() and list slice notation to get the RGB components from the hexadecimal string.
- Use tuple() to convert the resulting list to a tuple.
Sample Solution:
Python Code:
# Define a function to convert a hexadecimal color code to an RGB tuple
def hex_to_rgb(hex):
# Use a generator expression to convert pairs of hexadecimal digits to integers and create a tuple
return tuple(int(hex[i:i+2], 16) for i in (0, 2, 4))
# Test the function with different hexadecimal color codes and print the results
print(hex_to_rgb('FFA501'))
print(hex_to_rgb('FFFFFF'))
print(hex_to_rgb('000000'))
print(hex_to_rgb('FF0000'))
print(hex_to_rgb('000080'))
print(hex_to_rgb('C0C0C0'))
Sample Output:
(255, 165, 1) (255, 255, 255) (0, 0, 0) (255, 0, 0) (0, 0, 128) (192, 192, 192)
Flowchart:
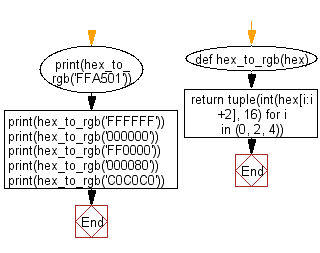
Python Code Editor:
Previous: Write a Python program to extract numbers from a given string.
Next: Write a Python program to convert the values of RGB components to a hexadecimal color code.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics