Python: Convert the values of RGB components to a hexadecimal color code
RGB to hex color code.
Write a Python program to convert the values of RGB components to a hexadecimal color code.
Create a placeholder for a zero-padded hexadecimal value using '{:02X}' and copy it three times.
Use str.format() on the resulting string to replace the placeholders with the given values.
Sample Solution:
Python Code:
# Define a function to convert RGB values to a hexadecimal color code
def rgb_to_hex(r, g, b):
# Use string formatting to convert the RGB values to a hexadecimal color code
return ('{:02X}' * 3).format(r, g, b)
# Test the function with different RGB values and print the results
print(rgb_to_hex(255, 165, 1))
print(rgb_to_hex(255, 255, 255))
print(rgb_to_hex(0, 0, 0))
print(rgb_to_hex(0, 0, 128))
print(rgb_to_hex(192, 192, 192))
Sample Output:
FFA501 FFFFFF 000000 000080 C0C0C0
Flowchart:
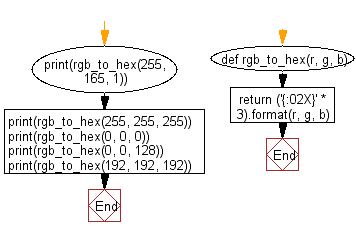
Python Code Editor:
Previous: Write a Python program to convert a hexadecimal color code to a tuple of integers corresponding to its RGB components.
Next: Write a Python program to convert a given string to camelcase.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics