Python: Convert a given string to Camelcase
Python String: Exercise-96 with Solution
From Wikipedia,
Camel case (sometimes stylized as camelCase or CamelCase; also known as camel caps or more formally as medial capitals) is the practice of writing phrases without spaces or punctuation, indicating the separation of words with a single capitalized letter, and the first word starting with either case.
Write a Python program to convert a given string to Camelcase.
- Use re.sub() to replace any - or _ with a space, using the regexp r"(_|-)+".
- Use str.title() to capitalize the first letter of each word and convert the rest to lowercase.
- Finally, use str.replace() to remove spaces between words.
Sample Solution:
Python Code:
# Import the 'sub' function from the 're' module for regular expression substitution
from re import sub
# Define a function to convert a string to camel case
def camel_case(s):
# Use regular expression substitution to replace underscores and hyphens with spaces,
# then title case the string (capitalize the first letter of each word), and remove spaces
s = sub(r"(_|-)+", " ", s).title().replace(" ", "")
# Join the string, ensuring the first letter is lowercase
return ''.join([s[0].lower(), s[1:]])
# Test the function with different input strings and print the results
print(camel_case('JavaScript'))
print(camel_case('Foo-Bar'))
print(camel_case('foo_bar'))
print(camel_case('--foo.bar'))
print(camel_case('Foo-BAR'))
print(camel_case('fooBAR'))
print(camel_case('foo bar'))
Sample Output:
javascript fooBar fooBar foo.Bar fooBar foobar fooBar
Flowchart:
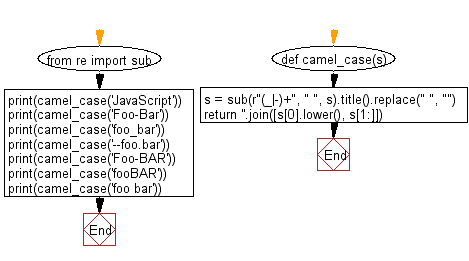
Python Code Editor:
Previous: Write a Python program to convert the values of RGB components to a hexadecimal color code.
Next: Write a Python program to convert a given string to snake case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/string/python-data-type-string-exercise-96.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics