Python Multi-threading and Concurrency: Concurrent even and odd number printing
Python Multi-threading: Exercise-3 with Solution
Write a Python program that creates two threads to find and print even and odd numbers from 30 to 50.
Sample Solution:
Python Code:
import threading
def print_even_numbers():
print("List of even numbers:")
for i in range(30, 51, 2):
print(i, end = " ")
def print_odd_numbers():
print("\nList of odd numbers:")
for i in range(31, 51, 2):
print(i, end = " ")
# Create threads for printing even and odd numbers
even_thread = threading.Thread(target=print_even_numbers)
odd_thread = threading.Thread(target=print_odd_numbers)
# Start the threads
even_thread.start()
odd_thread.start()
# Wait for the threads to complete
even_thread.join()
odd_thread.join()
Sample Output:
List of even numbers: 30 32 34 36 38 40 42 44 46 48 50 List of odd numbers: 31 33 35 37 39 41 43 45 47 49
Explanation:
In the above exercise,
- We define two functions: "print_even_numbers()" and "print_odd_numbers()".
- The "print_even_numbers()" function uses a loop to print even numbers from 30 to 50, incrementing by 2.
- The "print_odd_numbers()" function does the same for odd numbers, starting at 31 and incrementing by 2.
- Create two threads, "even_thread" and "odd_thread", targeting their respective functions.
- Start the threads using the start() method, which initiates their parallel execution.
- Finally, we use the join() method to wait for threads to complete.
Flowchart:
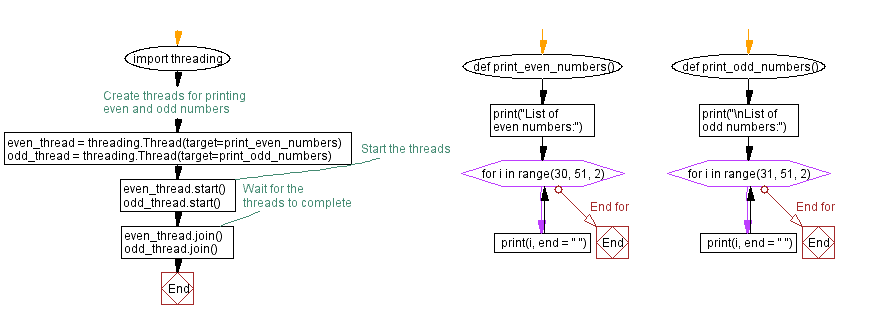
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Concurrent file downloading.
Next: Multi-threaded factorial calculation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/threading/python-multi-threading-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics