Build a simple calculator in Python using Tkinter
Python tkinter Basic: Exercise-13 with Solution
Write a Python program to design a simple calculator application using Tkinter with buttons for numbers and arithmetic operations.
Sample Solution:
Python Code:
import tkinter as tk
# Function to update the display
def update_display(value):
current_text = display_var.get()
if current_text == "0":
display_var.set(value)
else:
display_var.set(current_text + value)
# Function to clear the display
def clear_display():
display_var.set("0")
# Function to evaluate the expression and display the result
def calculate_result():
try:
result = eval(display_var.get())
display_var.set(result)
except Exception as e:
display_var.set("Error")
# Create the main window
parent = tk.Tk()
parent.title("Calculator")
# Create a variable to store the current display value
display_var = tk.StringVar()
display_var.set("0")
# Create the display label
display_label = tk.Label(parent, textvariable=display_var, font=("Arial", 24), anchor="e", bg="lightgray", padx=10, pady=10)
display_label.grid(row=0, column=0, columnspan=4)
# Define the button layout
button_layout = [
("7", 1, 0), ("8", 1, 1), ("9", 1, 2), ("/", 1, 3),
("4", 2, 0), ("5", 2, 1), ("6", 2, 2), ("*", 2, 3),
("1", 3, 0), ("2", 3, 1), ("3", 3, 2), ("-", 3, 3),
("0", 4, 0), (".", 4, 1), ("=", 4, 2), ("+", 4, 3),
]
# Create and place the buttons
for (text, row, col) in button_layout:
button = tk.Button(parent, text=text, padx=20, pady=20, font=("Arial", 18),
command=lambda t=text: update_display(t) if t != "=" else calculate_result())
button.grid(row=row, column=col)
# Create a Clear button
clear_button = tk.Button(parent, text="C", padx=20, pady=20, font=("Arial", 18), command=clear_display)
clear_button.grid(row=5, column=0, columnspan=3)
# Start the Tkinter event loop
parent.mainloop()
In the exercise above, we create a simple calculator GUI with buttons for numbers (0-9), arithmetic operations (+, -, *, /), a decimal point, an equals (=) button to calculate the result, and a clear (C) button to clear the display.
Note: Click the buttons to input numbers and perform arithmetic operations. The result will be displayed on the top label.
Sample Output:
Flowchart:
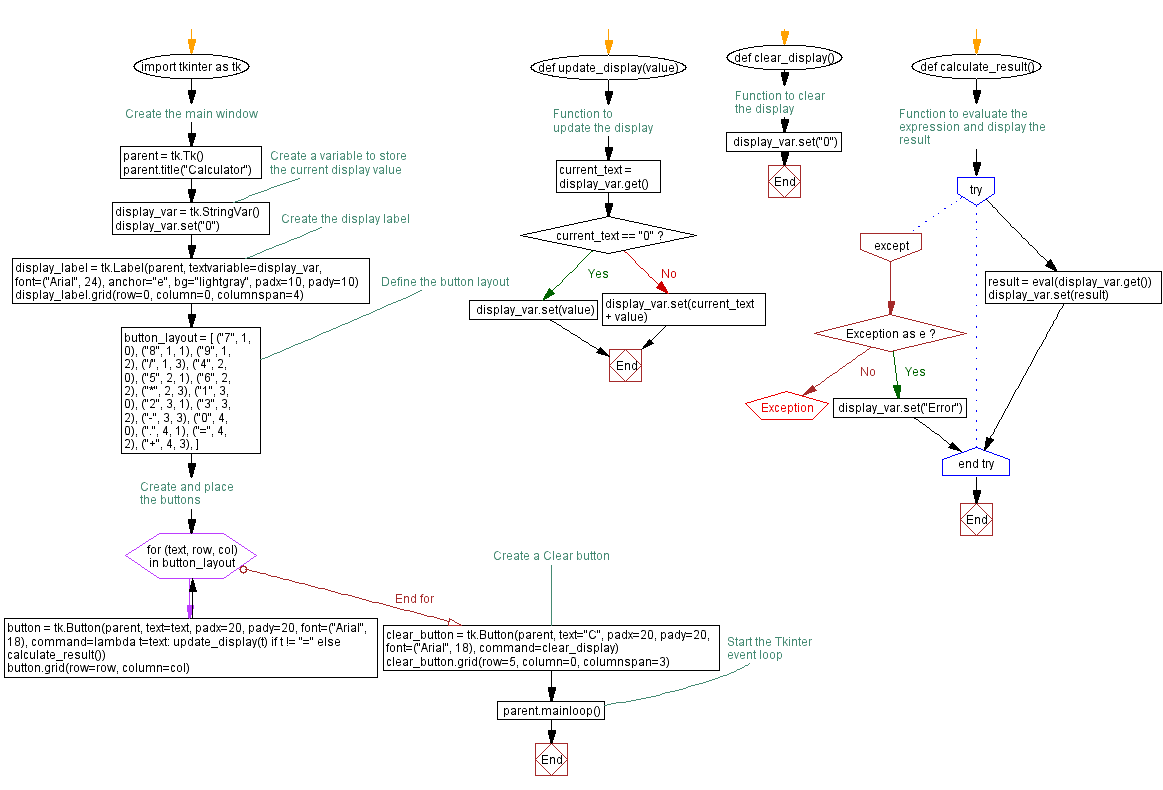
Python Code Editor:
Previous: Create a Python GUI window with custom background color.
Next: Create a digital clock in Python using Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/tkinter/python-tkinter-basic-exercise-13.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics