Create a temperature converter in Python using Tkinter
Write a Python program that implements a temperature converter application using Tkinter, allowing users to convert between Celsius and Fahrenheit.
Sample Solution:
Python Code:
import tkinter as tk
# Function to convert from Celsius to Fahrenheit
def celsius_to_fahrenheit():
try:
celsius = float(celsius_entry.get())
fahrenheit = (celsius * 9/5) + 32
result_label.config(text=f"{celsius:.2f}°C = {fahrenheit:.2f}°F")
except ValueError:
result_label.config(text="Invalid input")
# Function to convert from Fahrenheit to Celsius
def fahrenheit_to_celsius():
try:
fahrenheit = float(fahrenheit_entry.get())
celsius = (fahrenheit - 32) * 5/9
result_label.config(text=f"{fahrenheit:.2f}°F = {celsius:.2f}°C")
except ValueError:
result_label.config(text="Invalid input")
# Create the main window
parent = tk.Tk()
parent.title("Temperature Converter")
# Celsius to Fahrenheit Conversion
celsius_label = tk.Label(parent, text="Input Celsius:")
celsius_label.grid(row=0, column=0)
celsius_entry = tk.Entry(parent)
celsius_entry.grid(row=0, column=1)
c_to_f_button = tk.Button(parent, text="Convert to Fahrenheit", command=celsius_to_fahrenheit)
c_to_f_button.grid(row=0, column=2)
# Fahrenheit to Celsius Conversion
fahrenheit_label = tk.Label(parent, text="Input Fahrenheit:")
fahrenheit_label.grid(row=1, column=0)
fahrenheit_entry = tk.Entry(parent)
fahrenheit_entry.grid(row=1, column=1)
f_to_c_button = tk.Button(parent, text="Convert to Celsius", command=fahrenheit_to_celsius)
f_to_c_button.grid(row=1, column=2)
# Display the result
result_label = tk.Label(parent, text="", font=("Helvetica", 14))
result_label.grid(row=2, columnspan=3)
# Start the Tkinter event loop
parent.mainloop()
Explanation:
In the exercise above -
- Temperature values can be entered in Celsius or Fahrenheit.
- The result label will appear when they click the corresponding conversion button.
- A ValueError exception is thrown when invalid input is received, and the result label is displayed with the text "Invalid input".
- As a result of the Tkinter event loop (root.mainloop()), the GUI is kept running and responsive to user input.
Sample Output:
Flowchart:
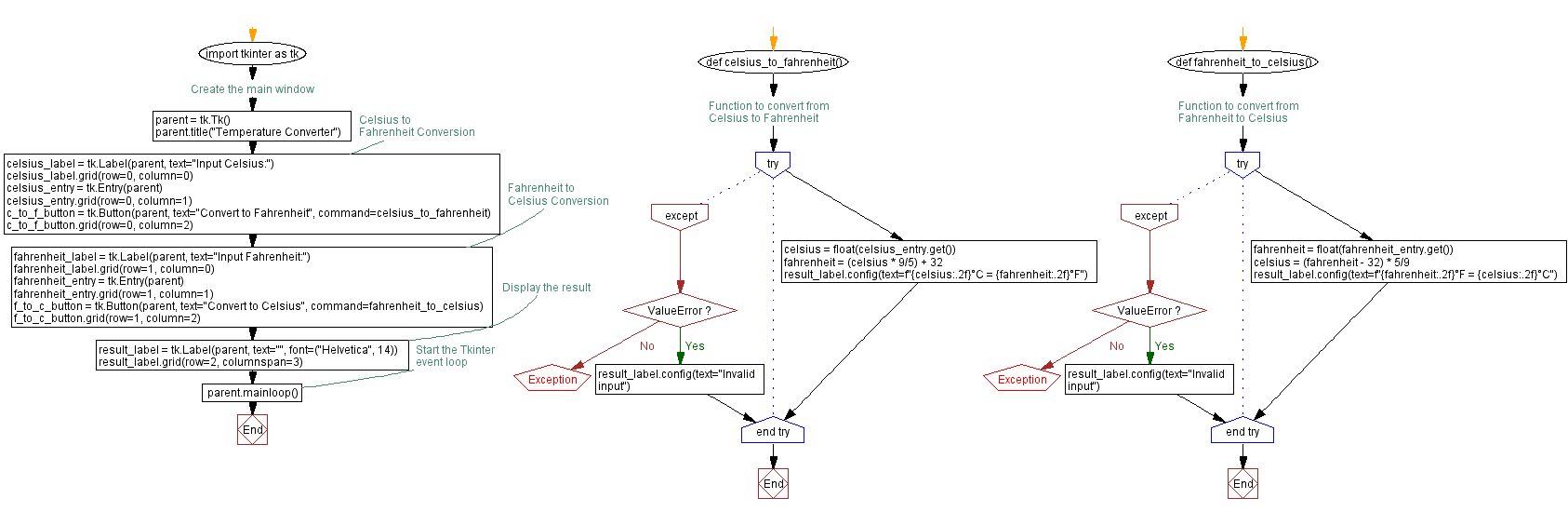
Python Code Editor:
Previous: Create a digital clock in Python using Tkinter.
Next: Create a login form in Python with Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.