Create interactive tooltips in a Python Tkinter window
Python tkinter Basic: Exercise-17 with Solution
Write a Python GUI program to add tooltips to buttons and labels in a Tkinter window.
Sample Solution:
Python Code:
import tkinter as tk
# Function to show tooltips
def show_tooltip(event, tooltip_text):
tooltip.geometry(f"+{event.x_parent + 10}+{event.y_parent + 10}") # Adjust tooltip position
tooltip_label.config(text=tooltip_text)
tooltip.deiconify()
# Function to hide tooltips
def hide_tooltip(event):
tooltip.withdraw()
# Create the main window
parent = tk.Tk()
parent.title("Tooltip Example")
# Create a button with a tooltip
button = tk.Button(parent, text="Button with Tooltip")
button.pack(padx=10, pady=10)
button.bind("<Enter>", lambda event, text="This is a button.": show_tooltip(event, text))
button.bind("<Leave>", hide_tooltip)
# Create a label with a tooltip
label = tk.Label(parent, text="Label with Tooltip")
label.pack(padx=10, pady=10)
label.bind("<Enter>", lambda event, text="This is a label.": show_tooltip(event, text))
label.bind("<Leave>", hide_tooltip)
# Create the tooltip window (hidden by default)
tooltip = tk.Toplevel(parent)
tooltip.withdraw()
tooltip_label = tk.Label(tooltip, text="", background="lightyellow", relief="solid", borderwidth=1)
tooltip_label.pack()
# Start the Tkinter event loop
parent.mainloop()
Explanation:
In the exercise above -
- We create a tooltip window (tooltip) using tk.Toplevel and initially hide it using tooltip.withdraw().
- The "show_tooltip()" function is modified to position and display the tooltip window when the mouse enters the widget. We use tooltip.deiconify() to show the tooltip.
- The "hide_tooltip()" function is used to hide the tooltip when the mouse leaves the widget by calling tooltip.withdraw().
Sample Output:
Flowchart:
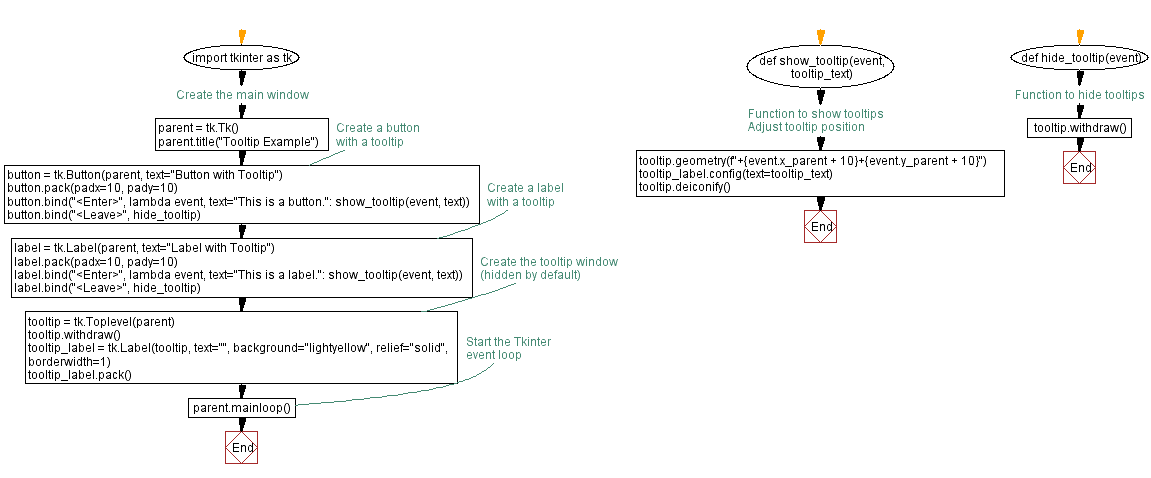
Python Code Editor:
Previous: Create a login form in Python with Tkinter.
Next: Create a Python GUI program to close a window.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/tkinter/python-tkinter-basic-exercise-17.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics