Python Tkinter Messagebox: Display messages
Write a Python program that displays messages in a messagebox using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import messagebox
# Create the main window
parent = tk.Tk()
parent.title("Messagebox Example")
# Function to display a message in a messagebox
def show_message():
messagebox.showinfo("Message", "Hello!")
# Create a button to trigger the messagebox
button = tk.Button(parent, text="Show Message", command=show_message)
button.pack()
# Start the Tkinter event loop
parent.mainloop()
In the exercise above, we create a “Tkinter” window with a button labeled "Show Message." When you click the button, it calls the "show_message()" function, which displays a messagebox with the title "Message" and the message "Hello!".
Sample Output:
Flowchart:
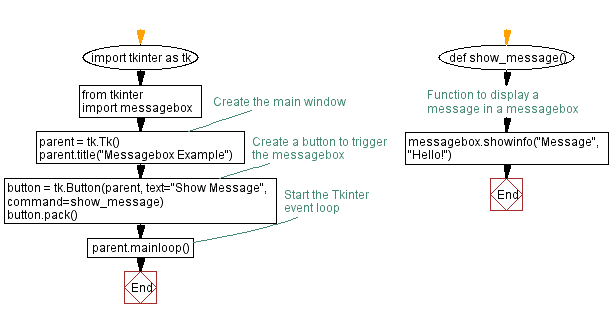
Go to:
Previous: Python Tkinter event handling: Button clicks.
Next: Customize labels and buttons in Python Tkinter.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.