Create an interactive geometric shape editor with Python and Tkinter
Write a Python program that creates an interactive map application with Tkinter and the Canvas widget. This will allow users to zoom in and out and pan across a map.
Sample Solution:
Python Code:
import tkinter as tk
import folium
from tkinter import ttk
from folium.plugins import MiniMap
# Define constants for the canvas size
CANVAS_WIDTH = 800
CANVAS_HEIGHT = 600
class MapApp:
def __init__(self, root):
self.root = root
self.root.title("Interactive Map")
# Create a Canvas widget
self.canvas = tk.Canvas(root, width=CANVAS_WIDTH, height=CANVAS_HEIGHT, bg="white")
self.canvas.pack()
# Create a Frame for the map
self.map_frame = ttk.Frame(root)
self.map_frame.place(x=0, y=0, width=CANVAS_WIDTH, height=CANVAS_HEIGHT)
# Create a map using Folium
self.m = folium.Map(location=[51.5074, -0.1278], zoom_start=10)
self.mini_map = MiniMap()
self.m.add_child(self.mini_map)
self.m.save('map.html')
# Load the map into the tkinter application
self.load_map()
def load_map(self):
# Use webbrowser to display the map HTML in a tkinter canvas
import webbrowser
webbrowser.open('map.html')
if __name__ == "__main__":
root = tk.Tk()
app = MapApp(root)
root.mainloop()
Explanation:
In the exercise above -
- Import following modules:
- tkinter: The standard Python interface to the Tk GUI toolkit.
- folium: A Python library for creating Leaflet.js maps.
- ttk from tkinter: Used for creating themed Tkinter widgets.
- MiniMap from folium.plugins: A plugin for "Folium" that adds a mini-map to the main map.
- Define constants for the canvas size (CANVAS_WIDTH and CANVAS_HEIGHT) with a width of 800 pixels and a height of 600 pixels.
- Create a class MapApp that encapsulates the map application:
- In the "init()" method:
- Initialize the main Tkinter root window (root) and set its title to "Interactive Map."
- Create a canvas widget (self.canvas) with the specified dimensions and a white background. This canvas will be used for displaying the map.
- Create a frame (self.map_frame) to hold the map. The frame is placed at the same position and size as the canvas.
- Create a Folium map (self.m) with an initial location (latitude and longitude) and zoom level. In this case, the location is set to London (51.5074, -0.1278), and the initial zoom level is 10.
- Add a MiniMap plugin (self.mini_map) to the main map.
- Save the map as an HTML file named 'map.html' using self.m.save('map.html').
- Define a load_map method:
- This method uses the "webbrowser" module to open the 'map.html' file in the default web browser. This is how the "Folium" map is displayed within the Tkinter application.
- In the if name == "__main__": block:
- Create the main Tkinter root window (root).
- Instantiate the "MapApp" class with the root as an argument, creating the map application.
- Start the Tkinter main event loop with "root.mainloop()".
Output:
Flowchart:
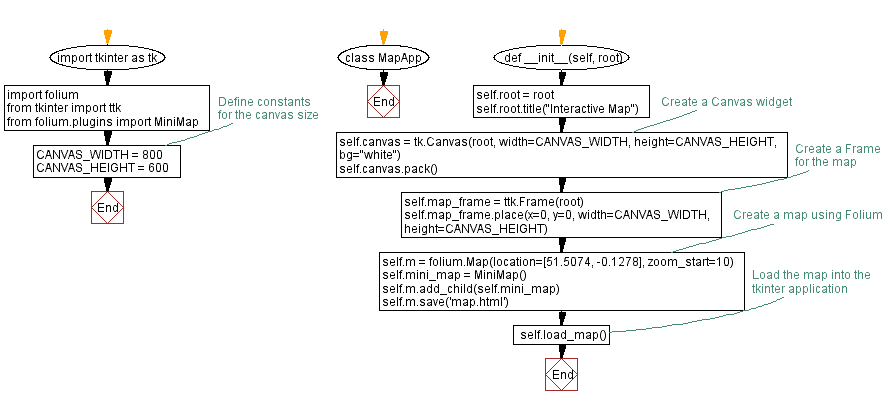
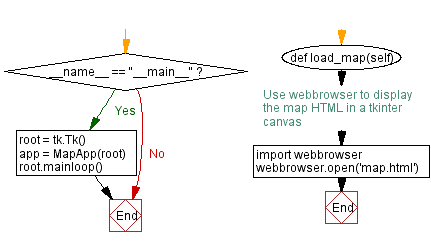
Python Code Editor:
Previous: Python Tkinter canvas shape editor: Drawing and manipulating shapes.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics