Creating a custom color picker in Python with Tkinter
Write a Python program to create a custom color picker widget that allows users to select colors from a custom color palette using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import colorchooser
class CustomColorPicker:
def __init__(self, root):
self.root = root
self.root.title("Custom Color Picker")
# Custom color palette
self.colors = ["#FF0000", "#00FF00", "#0000FF", "#FFFF00", "#FF00FF", "#00FFFF"]
# Create buttons for each color
self.color_buttons = []
for color in self.colors:
button = tk.Button(root, bg=color, width=4, height=2, command=lambda c=color: self.pick_color(c))
button.pack(side=tk.LEFT, padx=5, pady=5)
self.color_buttons.append(button)
def pick_color(self, color):
chosen_color = colorchooser.askcolor(initialcolor=color, parent=self.root, title="Select Color")
if chosen_color[1]:
print(f"Selected color: {chosen_color[1]}")
if __name__ == "__main__":
root = tk.Tk()
app = CustomColorPicker(root)
root.mainloop()
Explanation:
In the exercise above -
- Create a CustomColorPicker class to manage custom color pickers.
- Define a custom color palette as a list of color codes (self.colors).
- Create buttons for each color in the custom palette and assign background colors accordingly.
- When a color button is clicked, the "pick_color()" method is called, which opens a color selection dialog using colorchooser.askcolor. On the console, the selected color is displayed.
Output:
Selected color: #00ff00 Selected color: #d70428 Selected color: #0000ff![]()
Flowchart:
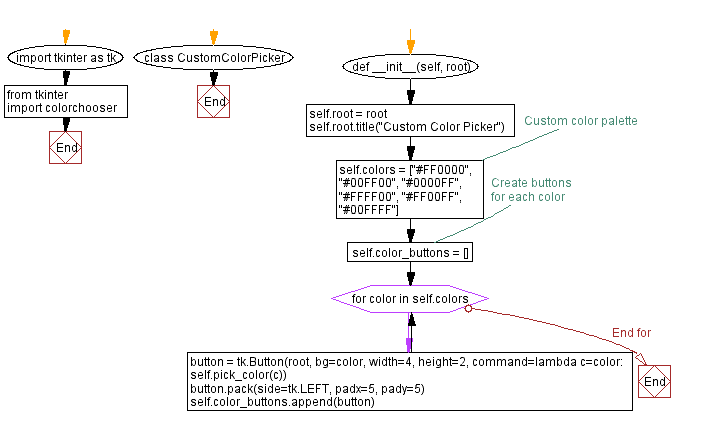
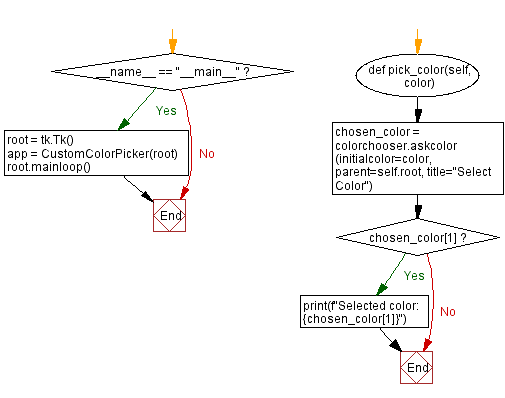
Go to:
Previous: Creating custom tooltips in Python with Tkinter.
Next: Creating a circular progress indicator in Python with Tkinter.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.