Creating a custom scrollbar widget in Python with Tkinter
Python Tkinter Custom Widgets and Themes: Exercise-12 with Solution
Write a Python program to build a custom scrollbar widget with a unique appearance, such as custom arrows or a background using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
class CustomScrollbar(tk.Frame):
def __init__(self, master, **kwargs):
super().__init__(master, **kwargs)
self.canvas = tk.Canvas(self, bg="lightgray")
self.scrollbar = tk.Scrollbar(self, orient="vertical", command=self.canvas.yview)
self.canvas.config(yscrollcommand=self.scrollbar.set)
self.scrollbar.pack(side="right", fill="y")
self.canvas.pack(side="left", fill="both", expand=True)
self.canvas.create_text(50, 50, text="Tkinter Exercises", fill="blue", font=("Arial", 14))
# Create custom arrow buttons
self.up_button = tk.Button(self, text="▲", command=self.scroll_up, font=("Arial", 12))
self.down_button = tk.Button(self, text="▼", command=self.scroll_down, font=("Arial", 12))
self.up_button.pack(side="top", fill="x")
self.down_button.pack(side="bottom", fill="x")
self.canvas.bind("", self.on_canvas_configure)
def scroll_up(self):
self.canvas.yview_scroll(-1, "units")
def scroll_down(self):
self.canvas.yview_scroll(1, "units")
def on_canvas_configure(self, event):
self.canvas.configure(scrollregion=self.canvas.bbox("all"))
if __name__ == "__main__":
root = tk.Tk()
root.title("Custom Scrollbar Example")
custom_scrollbar = CustomScrollbar(root)
custom_scrollbar.pack(fill="both", expand=True)
root.mainloop()
Explanation:
In the exercise above -
- Create a CustomScrollbar class that inherits from "tk.Frame".
- Inside the "CustomScrollbar" class, create a canvas widget with a custom background color and a vertical scrollbar widget.
- The scrollbar command option allows you to configure the canvas to be scrollable.
- Add custom arrow buttons ("▲" and "▼") to scroll up and down. These buttons use the scroll_up and scroll_down methods to scroll the canvas.
- A custom rectangle and text are included on the canvas for demonstration purposes.
- Bind the <Configure> event to the "on_canvas_configure()" method, which updates the canvas scroll region when the window is resized.
- In the if name == "__main__": block, we create a Tkinter window and add the 'CustomScrollbar' widget to it.
Output:
Flowchart:
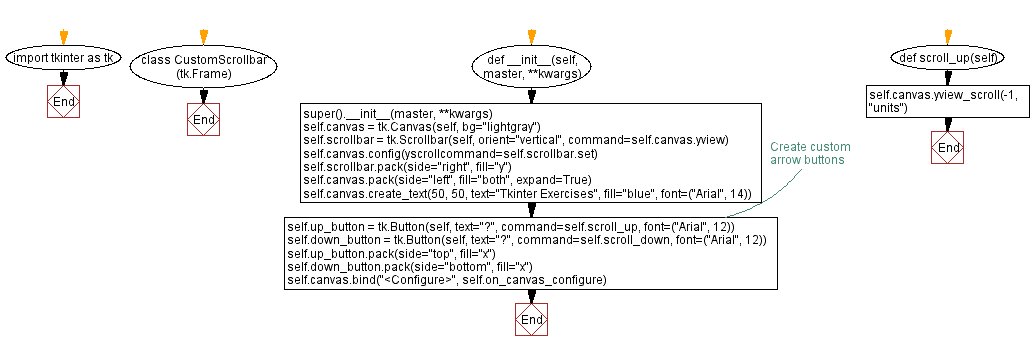
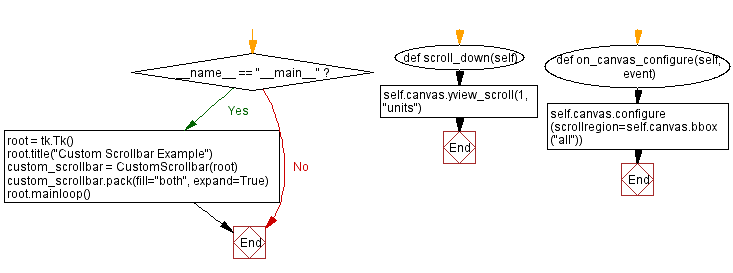
Python Code Editor:
Previous: Creating a circular progress indicator in Python with Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/tkinter/python-tkinter-custom-widgets-and-themes-exercise-12.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics