Customizing Tkinter entry Widgets with themes
Write a Python program that implements a themed "Entry" widget with a different background and border color using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
class ThemedEntry:
def __init__(self, master=None, **kwargs):
self.entry = tk.Entry(master, **kwargs)
self.entry.configure(
highlightthickness=2, # Set the border thickness
highlightcolor="red", # Set the border color
bg="lightblue" # Set the background color
)
if __name__ == "__main__":
root = tk.Tk()
root.title("Themed Entry Example")
themed_entry = ThemedEntry(root)
themed_entry.entry.pack(padx=20, pady=20)
root.mainloop()
Explanation:
In the exercise above -
- Import the "tkinter" library.
- Define a class called "ThemedEntry". This class represents a custom themed 'Entry' widget.
- In the init method of the "ThemedEntry" class, we initialize an Entry widget by calling tk.Entry(master, kwargs).
- We configure the appearance of the Entry widget using the .configure() method:
- highlightthickness=2: Sets the border thickness around the Entry widget to 2 pixels.
- highlightcolor="red": Sets the border color to red. You can replace "red" with any other valid color.
- bg="lightblue": Sets the background color of the Entry widget to light blue.
- In the if name == "__main__": block, we create the main application window (root) using tk.Tk() and set its title to "Themed Entry Example."
- Create an instance of the "ThemedEntry" class called "themed_entry".
- Finally, pack the 'entry' widget (contained within the "ThemedEntry" instance) inside the main window with padding ('padx' and 'pady') to ensure some spacing around the widget.
- Finally, start the Tkinter main loop with "root.mainloop()".
Output:
Flowchart:
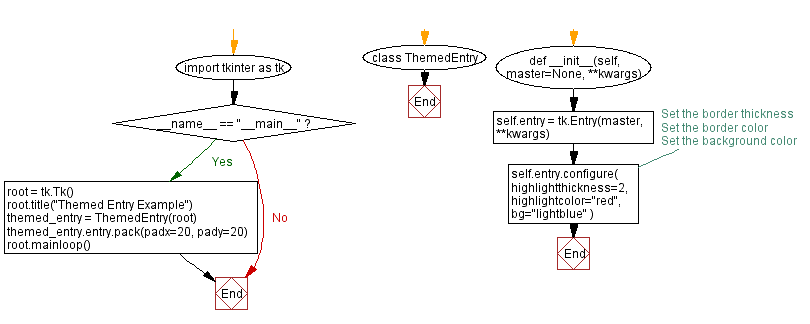
Go to:
Previous: Designing custom checkboxes in Python Tkinter.
Next: Customizing Tkinter radio buttons with distinct styles.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.