Creating a custom Tabbed interface in Python with Tkinter
Write a Python program that creates a custom tabbed interface widget that allows switching between multiple content panes using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import ttk
class CustomTabbedInterface:
def __init__(self, root):
self.root = root
self.root.title("Custom Tabbed Interface")
# Create a notebook-style tabbed interface
self.notebook = ttk.Notebook(root)
# Create content panes for each tab
self.tab1_content = ttk.Frame(self.notebook)
self.tab2_content = ttk.Frame(self.notebook)
# Add content panes to the notebook
self.notebook.add(self.tab1_content, text="Tab-1")
self.notebook.add(self.tab2_content, text="Tab-2")
# Add widgets to the content panes
self.label1 = ttk.Label(self.tab1_content, text="Content for Tab-1")
self.label2 = ttk.Label(self.tab2_content, text="Content for Tab-2")
self.label1.pack(padx=20, pady=20)
self.label2.pack(padx=20, pady=20)
# Pack the notebook
self.notebook.pack()
if __name__ == "__main__":
root = tk.Tk()
app = CustomTabbedInterface(root)
root.mainloop()
Explanation:
In the exercise above -
- Use the "ttk.Notebook" widget to create a tabbed interface.
- Two content panes ('tab1_content' and 'tab2_content') are added to the notebook.
- Add labels (label1 and label2) to each content pane to show different content for each tab.
- Tabs with labels "Tab-1" and "Tab-2" are automatically generated by the "ttk.Notebook" widget.
Output:
Flowchart:
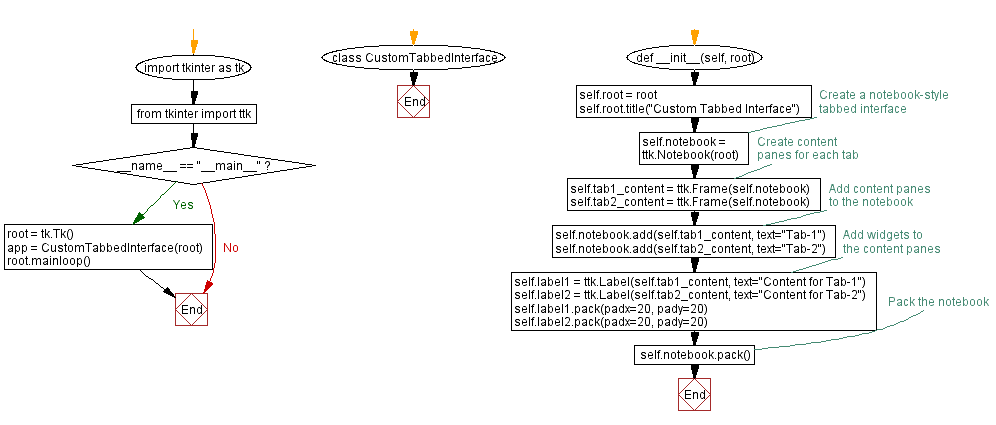
Python Code Editor:
Previous: Customizing Tkinter slider widget with a unique theme.
Next: Creating a custom combobox in Python with Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics