Python Tkinter: Creating an exit confirmation dialog
Python Tkinter Dialogs and File Handling: Exercise-2 with Solution
Write a Python program using Tkinter, that implements a confirmation dialog that asks the user if they want to save changes before exiting the application.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import messagebox
def confirm_exit():
response = messagebox.askyesnocancel("Confirm Exit", "Want to save changes before exiting?")
if response is None:
# User clicked "Cancel"
return
elif response:
# User clicked "Yes,"
save_changes()
# Close the application
root.destroy()
def save_changes():
# Implement your save changes logic here
messagebox.showinfo("Saved", "Saved successfully!")
root = tk.Tk()
root.title("Exit Example")
exit_button = tk.Button(root, text="Exit", command=confirm_exit)
exit_button.pack(padx=20, pady=20)
root.mainloop()
Explanation:
In the exercise above -
- import tkinter as tk - Import the required library for creating the GUI components.
- from tkinter import messagebox – Import messagebox for displaying the information dialog.
- Define a function confirm_exit(): It displays a messagebox with "Confirm Exit" as the title and asks the user whether they want to save changes before exiting. Depending on the user's response, it may call the “save_changes()” function and then close the application using root.destroy().
- Define a function save_changes(): Here we implement logic to save changes. It shows a messagebox with a "Saved" message for demonstration purposes.
- root = tk.Tk() - Create the main Tkinter window.
- exit_button = tk.Button(root, text="Exit", command=confirm_exit) - Create an "Exit" button (exit_button) that, when clicked, calls the “confirm_exit()” function.
- exit_button.pack(padx=20, pady=20) - Use the pack() method to display the button with some padding around it.
- root.mainloop() - The main event loop, root.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
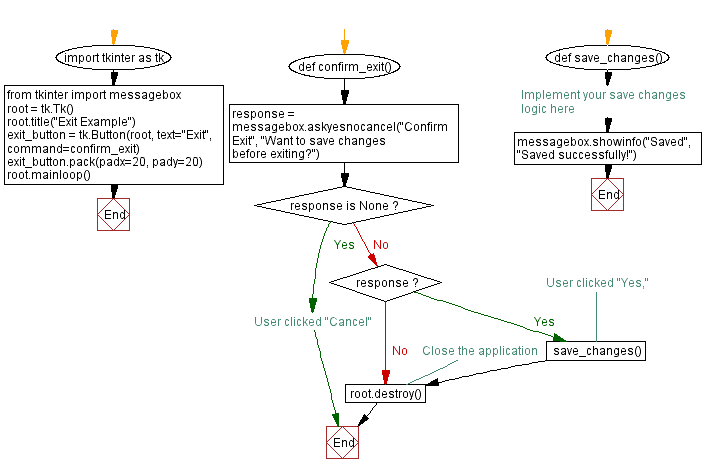
Python Code Editor:
Previous: Creating a simple information dialog.
Next: Opening a file dialog for file selection.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/tkinter/python-tkinter-dialogs-and-file-handling-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics